C# Tutorial
C# examples, c# assignment operators, assignment operators.
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
Try it Yourself »
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |


COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
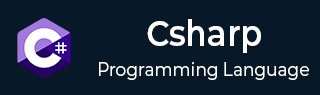
- C# Basic Tutorial
- C# - Overview
- C# - Environment
- C# - Program Structure
- C# - Basic Syntax
- C# - Data Types
- C# - Type Conversion
- C# - Variables
- C# - Constants
- C# - Operators
- C# - Decision Making
- C# - Encapsulation
- C# - Methods
- C# - Nullables
- C# - Arrays
- C# - Strings
- C# - Structure
- C# - Classes
- C# - Inheritance
- C# - Polymorphism
- C# - Operator Overloading
- C# - Interfaces
- C# - Namespaces
- C# - Preprocessor Directives
- C# - Regular Expressions
- C# - Exception Handling
- C# - File I/O
- C# Advanced Tutorial
- C# - Attributes
- C# - Reflection
- C# - Properties
- C# - Indexers
- C# - Delegates
- C# - Events
- C# - Collections
- C# - Generics
- C# - Anonymous Methods
- C# - Unsafe Codes
- C# - Multithreading
- C# Useful Resources
- C# - Questions and Answers
- C# - Quick Guide
- C# - Useful Resources
- C# - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
C# - Assignment Operators
There are following assignment operators supported by C# −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator, Assigns values from right side operands to left side operand | C = A + B assigns value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
The following example demonstrates all the assignment operators available in C# −
When the above code is compiled and executed, it produces the following result −
To Continue Learning Please Login
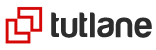
C# Assignment Operators with Examples
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand.
For example, we can declare and assign a value to the variable using the assignment operator ( = ) like as shown below.
If you observe the above sample, we defined a variable called “ a ” and assigned a new value using an assignment operator ( = ) based on our requirements.
The following table lists the different types of operators available in c# assignment operators.
Operator | Name | Description | Example |
---|---|---|---|
= | Equal to | It is used to assign the values to variables. | int a; a = 10 |
+= | Addition Assignment | It performs the addition of left and right operands and assigns a result to the left operand. | a += 10 is equals to a = a + 10 |
-= | Subtraction Assignment | It performs the subtraction of left and right operands and assigns a result to the left operand. | a -= 10 is equals to a = a - 10 |
*= | Multiplication Assignment | It performs the multiplication of left and right operands and assigns a result to the left operand. | a *= 10 is equals to a = a * 10 |
/= | Division Assignment | It performs the division of left and right operands and assigns a result to the left operand. | a /= 10 is equals to a = a / 10 |
%= | Modulo Assignment | It performs the modulo operation on two operands and assigns a result to the left operand. | a %= 10 is equals to a = a % 10 |
&= | Bitwise AND Assignment | It performs the Bitwise AND operation on two operands and assigns a result to the left operand. | a &= 10 is equals to a = a & 10 |
|= | Bitwise OR Assignment | It performs the Bitwise OR operation on two operands and assigns a result to the left operand. | a |= 10 is equals to a = a | 10 |
^= | Bitwise Exclusive OR Assignment | It performs the Bitwise XOR operation on two operands and assigns a result to the left operand. | a ^= 10 is equals to a = a ^ 10 |
>>= | Right Shift Assignment | It moves the left operand bit values to the right based on the number of positions specified by the second operand. | a >>= 2 is equals to a = a >> 2 |
<<= | Left Shift Assignment | It moves the left operand bit values to the left based on the number of positions specified by the second operand. | a <<= 2 is equals to a = a << 2 |
C# Assignment Operators Example
Following is the example of using assignment Operators in the c# programming language.
If you observe the above example, we defined a variable or operand “ x ” and assigning new values to that variable by using assignment operators in the c# programming language.
Output of C# Assignment Operators Example
When we execute the above c# program, we will get the result as shown below.
This is how we can use assignment operators in c# to assign new values to the variable based on our requirements.
Table of Contents
- Assignment Operators in C# with Examples
- C# Assignment Operator Example
- Output of C# Assignment Operator Example
C# Assignment Operators
Symbol | Operation | Example |
---|---|---|
+= | Plus Equal To | x+=15 is x=x+15 |
-= | Minus Equal To | x- =15 is x=x-15 |
*= | Multiply Equal To | x*=16 is x=x+16 |
%= | Modulus Equal To | x%=15 is x=x%15 |
/= | Divide Equal To | x/=16 is x=x/16 |
C# Assignment Operators Example
Operators are used to perform various operations on variables and values.
The following code snippet uses the assignment operator, = , to set myVariable to the value of num1 and num2 with an arithmetic operator operating on them. For example, if operator represented * , myVariable would be assigned a value of num1 * num2 .
Operators can be organized into the following groups:
- Arithmetic operators for performing traditional math evaluations.
- Assignment operators for assigning values to variables.
- Comparison operators for comparing two values.
- Logical operators for combining Boolean values.
- Bitwise operators for manipulating the bits of a number.
Arithmetic Operators
C# has the following arithmetic operators:
- Addition, + , returns the sum of two numbers.
- Subtraction, - , returns the difference between two numbers.
- Multiplication, * , returns the product of two numbers.
- Division, / , returns the quotient of two numbers.
- Modulus, % , returns the remainder of one number divided by another.
The ones above operate on two values. C# also has two unary operators:
- Increment, ++ , which increments its single operand by one.
- Decrement, -- , which decrements its single operand by one.
Unlike the other arithmetic operators, the increment and decrement operators change the value of their operand as well as return a value. They also return different results depending on if they precede or follow the operand. Preceding the operand returns the value of the operand before the operation. Following the operand returns the value of the operand after the operation.
Logical Operators
C# has the following logical operators:
- The & (and) operator returns true if both operands are true .
- The | (or) operator returns true if either operand is true .
- The ^ (xor) operator returns true if only one of its operands are true
- The ! (not) operator returns true if its single operand is false .
Note: & , | , and ^ are logical operators when the operands are bool types. When the operands are numbers they perform bitwise operations. See Bitwise Operators below.
The above operators always evaluate both operands. There are also these conditional “short circuiting” operators:
- The && (conditional and) operator returns true if both operands are true . If the first operand is false , the second operand is not evaluated.
- The || (conditional or) operator returns true if either operand is true . If the first operand is true the second operand is not evaluated.
Assignment Operators
C# includes the following assignment operators:
- The = operator assigns the value on the right to the variable on the left.
- The += operator updates a variable by incrementing its value and reassigning it.
- The -= operator updates a variable by decrementing its value and reassigning it.
- The *= operator updates a variable by multiplying its value and reassigning it.
- The /= operator updates a variable by dividing its value and reassigning it.
- The %= operator updates a variable by calculating its modulus against another value and reassigning it.
The assignment operators of the form op= , where op is a binary arithmetic operator, is a shorthand. The expression x = x op y; can be shortened to x op= y; . This compound assignment also works with the logical operators & , | and ^ .
- The ??= operator assigns the value on the right to the variable on the left if the variable on the left is null .
Comparison Operators
C# has the following comparison operators:
- Equal, == , for returning true if two values are equal.
- Not equal, != , for returning true if two values are not equal.
- Less than, < , for returning true if the left value is less than the right value.
- Less than or equal to, <= , for returning true if the left value is less than or equal to the right value.
- Greater than, > , for returning true if the left value is greater than the right value.
- Greater than or equal to, >= , for returning true if the left value is greater than or equal to the right value.
Note: for these comparison operators, if any operand is not a number ( Double.NaN or Single.NaN ) the result of the operation is false .
Bitwise Operators
C# has the following operators that perform operations on the individual bits of a number.
- Bitwise complement, ~ , inverts each bit in a number.
- Left-shift, << , shifts its left operand left by the number of bits specified in its right operand. New right positions are zero-filled.
- Right-shift, >> , shifts its left operand right by the number of bits specified in its right operand. For signed integers, left positions are filled with the value of the high-order bit. For unsigned integers, left positions are filled with zero.
- Unsigned right-shift, >>> , same as >> except left positions are always zero-filled.
- Logical AND, & , performs a bitwise logical AND of its operands.
- Logical OR, | , performs a bitwise logical OR on its operands.
- Logical XOR, ^ , performs a bitwise logical XOR on its operands.
All contributors
- StevenSwiniarski
Looking to contribute?
- Learn more about how to get involved.
- Edit this page on GitHub to fix an error or make an improvement.
- Submit feedback to let us know how we can improve Docs.
Learn C# on Codecademy
Computer science.
C# Assignment Operators
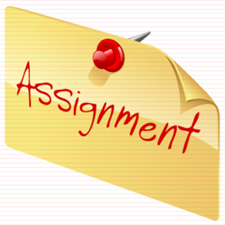
- What is C# Assignment Operators?
- How many types of assignment operators in C sharp?
- How to use assignment operator in program?
The C# assignment operator is generally suffix with arithmetic operators. The symbol of c sharp assignment operator is "=" without quotes. The assignment operator widely used with C# programming. Consider a simple example:
result=num1+num2;
In this example, the equal to (=) assignment operator assigns the value of num1 + num2 into result variable.
Various types of C# assignment operators are mentioned below:
Assignment Operators:
Assignment Operators | Usage | Examples |
---|---|---|
(Equal to) | result=5 | Assign the value 5 for result |
(Plus Equal to) | result+=5 | Same as result=result+5 |
(Minus Equal to) | result-=5 | Same as result=result-5 |
(Multiply Equal to) | result*=5 | Same as result=result*5 |
(Divide Equal to) | result/=5 | Same as result=result/5 |
(Modulus Equal to) | result%=5 | Same as result=result%5 |
In this chapter, you learned about different types of assignment operators in C# . You also learned how to use these assignment operators in a program. In next chapter, you will learn about Unary Operator in C# .
Share your thought
Complete Csharp Tutorial
Please support us by enabling ads on this page. Refresh YOU DON'T LIKE ADS, WE ALSO DON'T LIKE ADS! But we have to show ads on our site to keep it free and updated . We have to pay huge server costs, domain costs, CDN Costs, Developer Costs, Electricity and Internet Bill . Your little contribution will encourage us to regularly update this site.

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
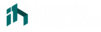
Home » .Net » C# Programs
C# Assignment Operators Example
C# example for assignment operators: Here, we are writing a C# program to demonstrate example of all assignment operators. By IncludeHelp Last updated : April 15, 2023
Assignment Operators
Assignment operators (Assignment ( = ) and compound assignments ( += , -+ , *= , /= , %= )) are used to assign the value or an expression's result to the left side variable, following are the set of assignment operators,
- "=" – it is used to assign value or an expression's result to the left side variable
- "+=" – it is used to add second operand to the existing operand's value and assigns it back (a+=b is equal to a=a+b)
- "-=" – it is used to subtract second operand from the existing operand's value and assigns it back (a-=b is equal to a=a-b)
- "/=" – it is used to divide second operand from the existing operand's value and assigns it back (a/=b is equal to a=a+b)
- "*=" – it is used to multiply second operand with the existing operand's value and assigns it back (a*=b is equal to a=a*b)
- "%=" – it is used to get the remainder by dividing second operand with the existing operand's value and assigns it back (a%=b is equal to a=a%b)
C# code to demonstrate the example of assignment operators
C# Basic Programs »
Related Programs
- C# program to print messages/text (program to print Hello world)
- C# program to demonstrate example of Console.Write() and Console.WriteLine()
- C# program to print a new line
- C# program to print backslash (\)
- C# program to demonstrate the example of New keyword
- C# program to print size of various data types
- C# program for type conversion from double to int
- C# program to convert various data type to string using ToString() method
- C# program to define various types of constants
- C# program to declare different types of variables, assign the values and print
- C# program to input and print an integer number
- C# program to demonstrate example of arithmetic operators
- C# program to demonstrate example of sizeof() operator
- C# program to demonstrate example of equal to and not equal to operators
- C# program to demonstrate example of relational operators
- C# program to demonstrate example of bitwise operators
- C# program to find the addition of two integer numbers
- C# program to swap two numbers with and without using third variable
- C# | print type, max and min value of various data types
- C# program to swap numbers using XOR operator
- C# program to find the magnitude of an integer number
- C# program to demonstrate the example of the left-shift operator
- C# program to demonstrate the example of the right shift operator
- C# program to read the grade of students and print the appropriate description of grade
- C# program to calculate the size of the area in square-feet based on specified length and width
- C# program to find the division of exponents of the same base
- C# program to demonstrate the example goto statement
- C# program to print a message without using the WriteLine() method
- C# program to convert a binary number into a decimal number
- C# program to convert a decimal number into a binary number
- C# program to convert a decimal number into an octal number
- C# program to convert a hexadecimal number into a decimal number
- C# program to convert a decimal number into a hexadecimal number
- C# program to convert a meter into kilo-meter and vice versa
- C# program to convert a temperature from Celsius to Fahrenheit
- C# program to convert a temperature from Fahrenheit into Celsius
- C# program to create gray code
- C# program to change the case of entered character
- C# program to convert entered days into years, weeks, and days
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
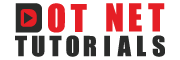
Operators in C#
Back to: C#.NET Tutorials For Beginners and Professionals
Operators in C# with Examples
In this article, I am going to discuss Operators in C# with Examples. Please read our previous article, where we discussed Variables in C# with Examples. The Operators are the foundation of any programming language. Thus, the functionality of the C# language is incomplete without the use of operators. At the end of this article, you will understand what are Operators and when, and how to use them in C# Application with examples.
What are Operators in C#?
Operators in C# are symbols that are used to perform operations on operands. For example, consider the expression 2 + 3 = 5 , here 2 and 3 are operands , and + and = are called operators . So, the Operators in C# are used to manipulate the variables and values in a program.
int x = 10, y = 20; int result1 = x + y; //Operator Manipulating Variables, where x and y are variables and + is operator int result2 = 10 + 20; //Operator Manipulating Values, where 10 and 20 are value and + is operator
Note: In the above example, x, y, 10, and 20 are called Operands. So, the operand may be variables or values.
Types of Operators in C#:
The Operators are classified based on the type of operations they perform on operands in C# language. They are as follows:
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Unary Operators or
- Ternary Operator or Conditional Operator
In C#, the Operators can also be categorized based on the Number of Operands:
- Unary Operator : The Operator that requires one operand (variable or value) to perform the operation is called Unary Operator.
- Binary Operator : Then Operator that requires two operands (variables or values) to perform the operation is called Binary Operator.
- Ternary Operator : The Operator that requires three operands (variables or values) to perform the operation is called Ternary Operator. The Ternary Operator is also called Conditional Operator.
For a better understanding of the different types of operators supported in C# Programming Language, please have a look at the below image.
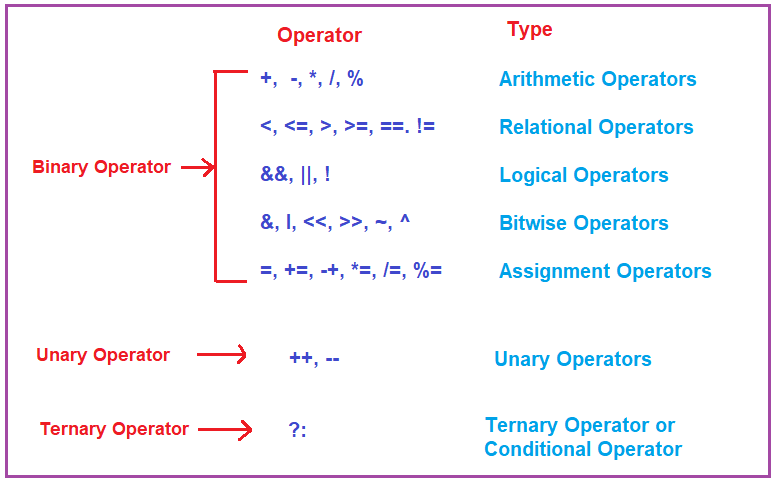
Arithmetic Operators in C#
The Arithmetic Operators in C# are used to perform arithmetic/mathematical operations like addition, subtraction, multiplication, division, etc. on operands. The following Operators are falling into this category.
Addition Operator (+): The + operator adds two operands. As this operator works with two operands, so, this + (plus) operator belongs to the category of the binary operator. The + Operator adds the left-hand side operand value with the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c = a+b; //15, Here, it will add the a and b operand values i.e. 10 + 5
Subtraction Operator (-): The – operator subtracts two operands. As this operator works with two operands, so, this – (minus) operator belongs to the category of the binary operator. The Minus Operator substracts the left-hand side operand value from the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c = a-b; //5, Here, it will subtract b from a i.e. 10 – 5
Multiplication Operator (*): The * (Multiply) operator multiplies two operands. As this operator works with two operands, so, this * (Multiply) operator belongs to the category of the binary operator. The Multiply Operator multiplies the left-hand side operand value with the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c=a*b; //50, Here, it will multiply a with b i.e. 10 * 5
Division Operator (/): The / (Division) operator divides two operands. As this operator works with two operands, so, this / (Division) operator belongs to the category of the binary operator. The Division Operator divides the left-hand side operand value with the right-hand side operand value and returns the result. For example: int a=10; int b=5; int c=a/b; //2, Here, it will divide 10 / 5
Modulus Operator (%): The % (Modulos) operator returns the remainder when the first operand is divided by the second. As this operator works with two operands, so, this % (Modulos) operator belongs to the category of the binary operator. For example: int a=10; int b=5; int c=a%b; //0, Here, it will divide 10 / 5 and it will return the remainder which is 0 in this case
Example to Understand Arithmetic Operators in C#:
In the below example, I am showing how to use Arithmetic Operators with Operand which are variables. Here, Num1 and Num2 are variables and all the Arithmetic Operators are working on these two variables.
In the following example, I am showing how to use Arithmetic Operators with Operand which are values. Here, 10 and 20 are values and all the Arithmetic Operators are working on these two values.
Note: The point that you need to remember is that the operator working on the operands and the operand may be variables, or values and can also be the combination of both.
Assignment Operators in C#:
The Assignment Operators in C# are used to assign a value to a variable. The left-hand side operand of the assignment operator is a variable and the right-hand side operand of the assignment operator can be a value or an expression that must return some value and that value is going to assign to the left-hand side variable.
The most important point that you need to keep in mind is that the value on the right-hand side must be of the same data type as the variable on the left-hand side else you will get a compile-time error. The different Types of Assignment Operators supported in the C# language are as follows:
Simple Assignment (=):
This operator is used to assign the value of the right-hand side operand to the left-hand side operand i.e. to a variable. For example: int a=10; int b=20; char ch = ‘a’; a=a+4; //(a=10+4) b=b-4; //(b=20-4)
Add Assignment (+=):
This operator is the combination of + and = operators. It is used to add the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=5; int b=6; a += b; //a=a+b; That means (a += b) can be written as (a = a + b)
Subtract Assignment (-=):
This operator is the combination of – and = operators. It is used to subtract the right-hand side operand value from the left-hand side operand value and then assign the result to the left-hand side variable. For example: int a=10; int b=5; a -= b; //a=a-b; That means (a -= b) can be written as (a = a – b)
Multiply Assignment (*=):
This operator is the combination of * and = operators. It is used to multiply the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=10; int b=5; a *= b; //a=a*b; That means (a *= b) can be written as (a = a * b)
Division Assignment (/=):
This operator is the combination of / and = operators. It is used to divide the left-hand side operand value with the right-hand side operand value and then assign the result to the left-hand side variable. For example: int a=10; int b=5; a /= b; //a=a/b; That means (a /= b) can be written as (a = a / b)
Modulus Assignment (%=):
This operator is the combination of % and = operators. It is used to divide the left-hand side operand value with the right-hand side operand value and then assigns the remainder of this division to the left-hand side variable. For example: int a=10; int b=5; a %= b; //a=a%b; That means (a %= b) can be written as (a = a % b)
Example to Understand Assignment Operators in C#:
Relational operators in c#:.
The Relational Operators in C# are also known as Comparison Operators. It determines the relationship between two operands and returns the Boolean results, i.e. true or false after the comparison. The Different Types of Relational Operators supported by C# are as follows.
Equal to (==):
This Operator is used to return true if the left-hand side operand value is equal to the right-hand side operand value. For example, 5==3 is evaluated to be false. So, this Equal to (==) operator will check whether the two given operand values are equal or not. If equal returns true else returns false.
Not Equal to (!=):
This Operator is used to return true if the left-hand side operand value is not equal to the right-hand side operand value. For example, 5!=3 is evaluated to be true. So, this Not Equal to (!=) operator will check whether the two given operand values are equal or not. If equal returns false else returns true.
Less than (<):
This Operator is used to return true if the left-hand side operand value is less than the right-hand side operand value. For example, 5<3 is evaluated to be false. So, this Less than (<) operator will check whether the first operand value is less than the second operand value or not. If so, returns true else returns false.
Less than or equal to (<=):
This Operator is used to return true if the left-hand side operand value is less than or equal to the right-hand side operand value. For example, 5<=5 is evaluated to be true. So. this Less than or equal to (<=) operator will check whether the first operand value is less than or equal to the second operand value. If so returns true else returns false.
Greater than (>):
This Operator is used to return true if the left-hand side operand value is greater than the right-hand side operand value. For example, 5>3 is evaluated to be true. So, this Greater than (>) operator will check whether the first operand value is greater than the second operand value. If so, returns true else return false.
Greater than or Equal to (>=):
This Operator is used to return true if the left-hand side operand value is greater than or equal to the right-hand side operand value. For example, 5>=5 is evaluated to be true. So, this Greater than or Equal to (>=) operator will check whether the first operand value is greater than or equal to the second operand value. If so, returns true else returns false.
Example to Understand Relational Operators in C#:
Logical operators in c#:.
The Logical Operators are mainly used in conditional statements and loops for evaluating a condition. These operators are going to work with boolean expressions. The different types of Logical Operators supported in C# are as follows:
Logical OR (||):
This operator is used to return true if either of the Boolean expressions is true. For example, false || true is evaluated to be true. That means the Logical OR (||) operator returns true when one (or both) of the conditions in the expression is satisfied. Otherwise, it will return false. For example, a || b returns true if either a or b is true. Also, it returns true when both a and b are true.
Logical AND (&&):
This operator is used to return true if all the Boolean Expressions are true. For example, false && true is evaluated to be false. That means the Logical AND (&&) operator returns true when both the conditions in the expression are satisfied. Otherwise, it will return false. For example, a && b return true only when both a and b are true.
Logical NOT (!):
This operator is used to return true if the condition in the expression is not satisfied. Otherwise, it will return false. For example, !a returns true if a is false.
Example to Understand Logical Operators in C#:
Bitwise operators in c#:.
The Bitwise Operators in C# perform bit-by-bit processing. They can be used with any of the integer (short, int, long, ushort, uint, ulong, byte) types. The different types of Bitwise Operators supported in C# are as follows.
Bitwise OR (|)
Bitwise OR operator is represented by |. This operator performs the bitwise OR operation on the corresponding bits of the two operands involved in the operation. If either of the bits is 1, it gives 1. If not, it gives 0. For example, int a=12, b=25; int result = a|b; //29 How? 12 Binary Number: 00001100 25 Binary Number: 00011001 Bitwise OR operation between 12 and 25: 00001100 00011001 ======== 00011101 (it is 29 in decimal) Note : If the operands are of type bool, the bitwise OR operation is equivalent to the logical OR operation between them.
Bitwise AND (&):
Bitwise OR operator is represented by &. This operator performs the bitwise AND operation on the corresponding bits of two operands involved in the operation. If both of the bits are 1, it gives 1. If either of the bits is not 1, it gives 0. For example, int a=12, b=25; int result = a&b; //8 How? 12 Binary Number: 00001100 25 Binary Number: 00011001 Bitwise AND operation between 12 and 25: 00001100 00011001 ======== 00001000 (it is 8 in decimal) Note : If the operands are of type bool, the bitwise AND operation is equivalent to the logical AND operation between them.
Bitwise XOR (^):
The bitwise OR operator is represented by ^. This operator performs a bitwise XOR operation on the corresponding bits of two operands. If the corresponding bits are different, it gives 1. If the corresponding bits are the same, it gives 0. For example, int a=12, b=25; int result = a^b; //21 How? 12 Binary Number: 00001100 25 Binary Number: 00011001 Bitwise AND operation between 12 and 25: 00001100 00011001 ======== 00010101 (it is 21 in decimal)
Example to Understand Bitwise Operators in C#:
In the above example, we are using BIT Wise Operators with integer data type and hence it performs the Bitwise Operations. But, if use BIT-wise Operators with boolean data types, then these bitwise operators AND, OR, and XOR behaves like Logical AND, and OR operations. For a better understanding, please have a look at the below example. In the below example, we are using the BIT-wise operators on boolean operands and hence they are going to perform the Logical AND, OR, and XOR Operations.
Note: The point that you need to remember while working with BIT-Wise Operator is that, depending on the operand on which they are working, the behavior is going to change. It means if they are working with integer operands, they will work like bitwise operators and return the result as an integer and if they are working with boolean operands, then work like logical operators and return the result as a boolean.

Unary Operators in C#:
The Unary Operators in C# need only one operand. They are used to increment or decrement a value. There are two types of Unary Operators. They are as follows:
- Increment operators (++): Example: (++x, x++)
- Decrement operators (–): Example: (–x, x–)
Increment Operator (++) in C# Language:
The Increment Operator (++) is a unary operator. It operates on a single operand only. Again, it is classified into two types:
- Post-Increment Operator
- Pre-Increment Operator
Post Increment Operators:
The Post Increment Operators are the operators that are used as a suffix to its variable. It is placed after the variable. For example, a++ will also increase the value of the variable a by 1.
Syntax: Variable++; Example: x++;
Pre-Increment Operators:
The Pre-Increment Operators are the operators which are used as a prefix to its variable. It is placed before the variable. For example, ++a will increase the value of the variable a by 1.
Syntax: ++Variable; Example: ++x;
Decrement Operators in C# Language:
The Decrement Operator (–) is a unary operator. It takes one value at a time. It is again classified into two types. They are as follows:
- Post Decrement Operator
- Pre-Decrement Operator
Post Decrement Operators:
The Post Decrement Operators are the operators that are used as a suffix to its variable. It is placed after the variable. For example, a– will also decrease the value of the variable a by 1.
Syntax: Variable–; Example: x–;
Pre-Decrement Operators:
The Pre-Decrement Operators are the operators that are a prefix to its variable. It is placed before the variable. For example, –a will decrease the value of the variable a by 1.
Syntax: –Variable; Example: — x;
Note: Increment Operator means to increment the value of the variable by 1 and Decrement Operator means to decrement the value of the variable by 1.
Example to Understand Increment Operators in C# Language:
Example to understand decrement operators in c# language:, five steps to understand how the unary operators works in c#.
I see, many of the students and developers getting confused when they use increment and decrement operators in an expression. To make you understand how exactly the unary ++ and — operators work in C#, we need to follow 5 simple steps. The steps are shown in the below diagram.
- Step 1: If there is some pre-increment or pre-decrement in the expression, that should execute first.
- Step 2: The second step is to substitute the values in the expression.
- Step 3: In the third step we need to evaluate the expression.
- Step 4: I n the fourth step Assignment needs to be performed.
- Step 5: The final step is to perform post-increment or post-decrement.
Now, if you have still doubt about the above five steps, then don’t worry we will see some examples to understand this step in a better way.
Example to Understand Increment and Decrement Operators in C# Language:
Let us see one complex example to understand this concept. Please have a look at the following example. Here, we are declaring three variables x, y, and z, and then evaluating the expression as z = x++ * –y; finally, we are printing the value of x, y, and z in the console.
Let us evaluate the expression z = x++ * –y; by following the above 5 steps:
- The First step is Pre-Increment or Pre-Decrement . Is there any pre-increment or pre-decrement in the expression? There is no pre-increment but there is a pre-decrement in the expression i.e. –y. So, execute that pre-decrement operator which will decrease the value of y by 1 i.e. now y becomes 19.
- The second step is Substitution . So, substitute the values of x and y. That means x will be substituted by 10 and y will be substituted by 19.
- The third step is Evaluation . So, evaluate the expression i.e. 10 * 19 = 190.
- The fourth step is the Assignment . So, assign the evaluated value to the given variable i.e. 190 will be assigned to z. So, now the z value becomes 190.
- The last step is Post-Increment and Post-Decrement . Is there any post-increment or post-decrement in the expression? There is no post-decrement but there is a post-increment in the expression i.e. x++. So, execute that post-increment which will increase the value of x by 1 i.e. x becomes 11.
So, when you will execute the above program it will print the x, y, and z values as 11, 19, and 190 respectively.
Note: It is not recommended by Microsoft to use the ++ or — operators inside a complex expression like the above example. The reason is if we use the ++ or — operator on the same variable multiple times in an expression, then we cannot predict the output. So, if you are just incrementing the value of a variable by 1 or decrementing the variable by 1, then in that scenario you need to use these Increment or Decrement Operators. One of the ideal scenarios where you need to use the increment or decrement operator is inside a loop. What is a loop, why loop, and what is a counter variable, we will discuss this in our upcoming articles, but now just have a look at the following example, where I am using the for loop and increment operator?
Ternary Operator in C#:
The Ternary Operator in C# is also known as the Conditional Operator ( ?: ). It is actually the shorthand of the if-else statement. It is called ternary because it has three operands or arguments. The first argument is a comparison argument, the second is the result of a true comparison, and the third is the result of a false comparison.
Syntax: Condition? first_expression : second_expression;
The above statement means that first, we need to evaluate the condition. If the condition is true the first_expression is executed and becomes the result and if the condition is false, the second_expression is executed and becomes the result.
Example to understand Ternary Operator in C#:
Output: Result = 20
In the next article, I am going to discuss Control Flow Statements in C# with Examples. Here, in this article, I try to explain Operators in C# with Examples and I hope you enjoy this Operators in C# article. I would like to have your feedback. Please post your feedback, question, or comments about this article.
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
3 thoughts on “Operators in C#”
Hi Sir.. just noticed.. plz correct result of ternary operator is as 20..
Very clear and concise. Never seen like this before.
VERY GOOD EXPLANATION
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Operators
Precedence and associativity of operators in python.
- Python Arithmetic Operators
- Difference between / vs. // operator in Python
- Python - Star or Asterisk operator ( * )
- What does the Double Star operator mean in Python?
- Division Operators in Python
- Modulo operator (%) in Python
- Python Logical Operators
- Python OR Operator
- Difference between 'and' and '&' in Python
- not Operator in Python | Boolean Logic
Ternary Operator in Python
- Python Bitwise Operators
Python Assignment Operators
Assignment operators in python.
- Walrus Operator in Python 3.8
- Increment += and Decrement -= Assignment Operators in Python
- Merging and Updating Dictionary Operators in Python 3.9
- New '=' Operator in Python3.8 f-string
Python Relational Operators
- Comparison Operators in Python
- Python NOT EQUAL operator
- Difference between == and is operator in Python
- Chaining comparison operators in Python
- Python Membership and Identity Operators
- Difference between != and is not operator in Python
In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators.
- OPERATORS: These are the special symbols. Eg- + , * , /, etc.
- OPERAND: It is the value on which the operator is applied.
Types of Operators in Python
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Identity Operators and Membership Operators
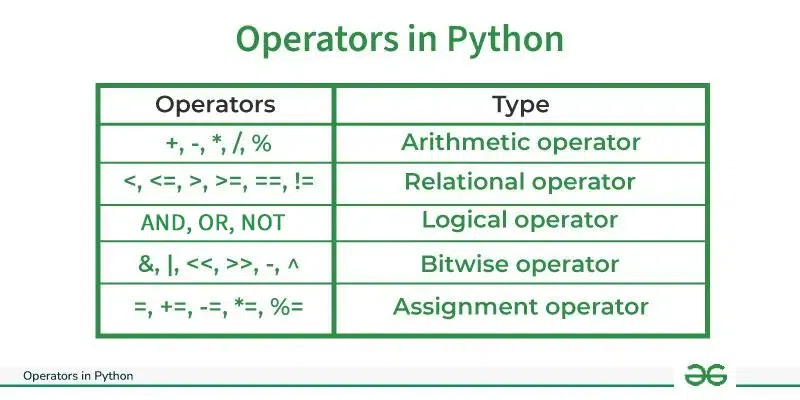
Arithmetic Operators in Python
Python Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication , and division .
In Python 3.x the result of division is a floating-point while in Python 2.x division of 2 integers was an integer. To obtain an integer result in Python 3.x floored (// integer) is used.
Operator | Description | Syntax |
---|---|---|
+ | Addition: adds two operands | x + y |
– | Subtraction: subtracts two operands | x – y |
* | Multiplication: multiplies two operands | x * y |
/ | Division (float): divides the first operand by the second | x / y |
// | Division (floor): divides the first operand by the second | x // y |
% | Modulus: returns the remainder when the first operand is divided by the second | x % y |
** | Power: Returns first raised to power second | x ** y |
Example of Arithmetic Operators in Python
Division operators.
In Python programming language Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient.
There are two types of division operators:
Float division
- Floor division
The quotient returned by this operator is always a float number, no matter if two numbers are integers. For example:
Example: The code performs division operations and prints the results. It demonstrates that both integer and floating-point divisions return accurate results. For example, ’10/2′ results in ‘5.0’ , and ‘-10/2’ results in ‘-5.0’ .
Integer division( Floor division)
The quotient returned by this operator is dependent on the argument being passed. If any of the numbers is float, it returns output in float. It is also known as Floor division because, if any number is negative, then the output will be floored. For example:
Example: The code demonstrates integer (floor) division operations using the // in Python operators . It provides results as follows: ’10//3′ equals ‘3’ , ‘-5//2’ equals ‘-3’ , ‘ 5.0//2′ equals ‘2.0’ , and ‘-5.0//2’ equals ‘-3.0’ . Integer division returns the largest integer less than or equal to the division result.
Precedence of Arithmetic Operators in Python
The precedence of Arithmetic Operators in Python is as follows:
- P – Parentheses
- E – Exponentiation
- M – Multiplication (Multiplication and division have the same precedence)
- D – Division
- A – Addition (Addition and subtraction have the same precedence)
- S – Subtraction
The modulus of Python operators helps us extract the last digit/s of a number. For example:
- x % 10 -> yields the last digit
- x % 100 -> yield last two digits
Arithmetic Operators With Addition, Subtraction, Multiplication, Modulo and Power
Here is an example showing how different Arithmetic Operators in Python work:
Example: The code performs basic arithmetic operations with the values of ‘a’ and ‘b’ . It adds (‘+’) , subtracts (‘-‘) , multiplies (‘*’) , computes the remainder (‘%’) , and raises a to the power of ‘b (**)’ . The results of these operations are printed.
Note: Refer to Differences between / and // for some interesting facts about these two Python operators.
Comparison of Python Operators
In Python Comparison of Relational operators compares the values. It either returns True or False according to the condition.
Operator | Description | Syntax |
---|---|---|
> | Greater than: True if the left operand is greater than the right | x > y |
< | Less than: True if the left operand is less than the right | x < y |
== | Equal to: True if both operands are equal | x == y |
!= | Not equal to – True if operands are not equal | x != y |
>= | Greater than or equal to True if the left operand is greater than or equal to the right | x >= y |
<= | Less than or equal to True if the left operand is less than or equal to the right | x <= y |
= is an assignment operator and == comparison operator.
Precedence of Comparison Operators in Python
In Python, the comparison operators have lower precedence than the arithmetic operators. All the operators within comparison operators have the same precedence order.
Example of Comparison Operators in Python
Let’s see an example of Comparison Operators in Python.
Example: The code compares the values of ‘a’ and ‘b’ using various comparison Python operators and prints the results. It checks if ‘a’ is greater than, less than, equal to, not equal to, greater than, or equal to, and less than or equal to ‘b’ .
Logical Operators in Python
Python Logical operators perform Logical AND , Logical OR , and Logical NOT operations. It is used to combine conditional statements.
Operator | Description | Syntax |
---|---|---|
and | Logical AND: True if both the operands are true | x and y |
or | Logical OR: True if either of the operands is true | x or y |
not | Logical NOT: True if the operand is false | not x |
Precedence of Logical Operators in Python
The precedence of Logical Operators in Python is as follows:
- Logical not
- logical and
Example of Logical Operators in Python
The following code shows how to implement Logical Operators in Python:
Example: The code performs logical operations with Boolean values. It checks if both ‘a’ and ‘b’ are true ( ‘and’ ), if at least one of them is true ( ‘or’ ), and negates the value of ‘a’ using ‘not’ . The results are printed accordingly.
Bitwise Operators in Python
Python Bitwise operators act on bits and perform bit-by-bit operations. These are used to operate on binary numbers.
Operator | Description | Syntax |
---|---|---|
& | Bitwise AND | x & y |
| | Bitwise OR | x | y |
~ | Bitwise NOT | ~x |
^ | Bitwise XOR | x ^ y |
>> | Bitwise right shift | x>> |
<< | Bitwise left shift | x<< |
Precedence of Bitwise Operators in Python
The precedence of Bitwise Operators in Python is as follows:
- Bitwise NOT
- Bitwise Shift
- Bitwise AND
- Bitwise XOR
Here is an example showing how Bitwise Operators in Python work:
Example: The code demonstrates various bitwise operations with the values of ‘a’ and ‘b’ . It performs bitwise AND (&) , OR (|) , NOT (~) , XOR (^) , right shift (>>) , and left shift (<<) operations and prints the results. These operations manipulate the binary representations of the numbers.
Python Assignment operators are used to assign values to the variables.
Operator | Description | Syntax |
---|---|---|
= | Assign the value of the right side of the expression to the left side operand | x = y + z |
+= | Add AND: Add right-side operand with left-side operand and then assign to left operand | a+=b a=a+b |
-= | Subtract AND: Subtract right operand from left operand and then assign to left operand | a-=b a=a-b |
*= | Multiply AND: Multiply right operand with left operand and then assign to left operand | a*=b a=a*b |
/= | Divide AND: Divide left operand with right operand and then assign to left operand | a/=b a=a/b |
%= | Modulus AND: Takes modulus using left and right operands and assign the result to left operand | a%=b a=a%b |
//= | Divide(floor) AND: Divide left operand with right operand and then assign the value(floor) to left operand | a//=b a=a//b |
**= | Exponent AND: Calculate exponent(raise power) value using operands and assign value to left operand | a**=b a=a**b |
&= | Performs Bitwise AND on operands and assign value to left operand | a&=b a=a&b |
|= | Performs Bitwise OR on operands and assign value to left operand | a|=b a=a|b |
^= | Performs Bitwise xOR on operands and assign value to left operand | a^=b a=a^b |
>>= | Performs Bitwise right shift on operands and assign value to left operand | a>>=b a=a>>b |
<<= | Performs Bitwise left shift on operands and assign value to left operand | a <<= b a= a << b |
Let’s see an example of Assignment Operators in Python.
Example: The code starts with ‘a’ and ‘b’ both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on ‘b’ . The results of each operation are printed, showing the impact of these operations on the value of ‘b’ .
Identity Operators in Python
In Python, is and is not are the identity operators both are used to check if two values are located on the same part of the memory. Two variables that are equal do not imply that they are identical.
Example Identity Operators in Python
Let’s see an example of Identity Operators in Python.
Example: The code uses identity operators to compare variables in Python. It checks if ‘a’ is not the same object as ‘b’ (which is true because they have different values) and if ‘a’ is the same object as ‘c’ (which is true because ‘c’ was assigned the value of ‘a’ ).
Membership Operators in Python
In Python, in and not in are the membership operators that are used to test whether a value or variable is in a sequence.
Examples of Membership Operators in Python
The following code shows how to implement Membership Operators in Python:
Example: The code checks for the presence of values ‘x’ and ‘y’ in the list. It prints whether or not each value is present in the list. ‘x’ is not in the list, and ‘y’ is present, as indicated by the printed messages. The code uses the ‘in’ and ‘not in’ Python operators to perform these checks.
in Python, Ternary operators also known as conditional expressions are operators that evaluate something based on a condition being true or false. It was added to Python in version 2.5.
It simply allows testing a condition in a single line replacing the multiline if-else making the code compact.
Syntax : [on_true] if [expression] else [on_false]
Examples of Ternary Operator in Python
The code assigns values to variables ‘a’ and ‘b’ (10 and 20, respectively). It then uses a conditional assignment to determine the smaller of the two values and assigns it to the variable ‘min’ . Finally, it prints the value of ‘min’ , which is 10 in this case.
In Python, Operator precedence and associativity determine the priorities of the operator.
Operator Precedence in Python
This is used in an expression with more than one operator with different precedence to determine which operation to perform first.
Let’s see an example of how Operator Precedence in Python works:
Example: The code first calculates and prints the value of the expression 10 + 20 * 30 , which is 610. Then, it checks a condition based on the values of the ‘name’ and ‘age’ variables. Since the name is “ Alex” and the condition is satisfied using the or operator, it prints “Hello! Welcome.”
Operator Associativity in Python
If an expression contains two or more operators with the same precedence then Operator Associativity is used to determine. It can either be Left to Right or from Right to Left.
The following code shows how Operator Associativity in Python works:
Example: The code showcases various mathematical operations. It calculates and prints the results of division and multiplication, addition and subtraction, subtraction within parentheses, and exponentiation. The code illustrates different mathematical calculations and their outcomes.
To try your knowledge of Python Operators, you can take out the quiz on Operators in Python .
Python Operator Exercise Questions
Below are two Exercise Questions on Python Operators. We have covered arithmetic operators and comparison operators in these exercise questions. For more exercises on Python Operators visit the page mentioned below.
Q1. Code to implement basic arithmetic operations on integers
Q2. Code to implement Comparison operations on integers
Explore more Exercises: Practice Exercise on Operators in Python
Please Login to comment...
Similar reads.
- python-basics
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
?: operator - the ternary conditional operator
- 11 contributors
The conditional operator ?: , also known as the ternary conditional operator, evaluates a Boolean expression and returns the result of one of the two expressions, depending on whether the Boolean expression evaluates to true or false , as the following example shows:
As the preceding example shows, the syntax for the conditional operator is as follows:
The condition expression must evaluate to true or false . If condition evaluates to true , the consequent expression is evaluated, and its result becomes the result of the operation. If condition evaluates to false , the alternative expression is evaluated, and its result becomes the result of the operation. Only consequent or alternative is evaluated. Conditional expressions are target-typed. That is, if a target type of a conditional expression is known, the types of consequent and alternative must be implicitly convertible to the target type, as the following example shows:
If a target type of a conditional expression is unknown (for example, when you use the var keyword) or the type of consequent and alternative must be the same or there must be an implicit conversion from one type to the other:
The conditional operator is right-associative, that is, an expression of the form
is evaluated as
You can use the following mnemonic device to remember how the conditional operator is evaluated:
Conditional ref expression
A conditional ref expression conditionally returns a variable reference, as the following example shows:
You can ref assign the result of a conditional ref expression, use it as a reference return or pass it as a ref , out , in , or ref readonly method parameter . You can also assign to the result of a conditional ref expression, as the preceding example shows.
The syntax for a conditional ref expression is as follows:
Like the conditional operator, a conditional ref expression evaluates only one of the two expressions: either consequent or alternative .
In a conditional ref expression, the type of consequent and alternative must be the same. Conditional ref expressions aren't target-typed.
Conditional operator and an if statement
Use of the conditional operator instead of an if statement might result in more concise code in cases when you need conditionally to compute a value. The following example demonstrates two ways to classify an integer as negative or nonnegative:
Operator overloadability
A user-defined type can't overload the conditional operator.
C# language specification
For more information, see the Conditional operator section of the C# language specification .
Specifications for newer features are:
- Target-typed conditional expression
- Simplify conditional expression (style rule IDE0075)
- C# operators and expressions
- if statement
- ?. and ?[] operators
- ?? and ??= operators
- ref keyword
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Bitwise assignment operators in C#
Operators like |= and &= work as bitwise operators on ints and longs...
But on a bool, it's a logical operation:
How do the ^= , &= and |= operators decide which manipulation to use when being applied to different data types?
- bit-manipulation
- 1 But on a bool, it's a logical operation: . Well, yes. But bools are single bits, and so a bit-wise OR is the same as a logical OR. – Rob ♦ Commented Nov 28, 2011 at 1:47
The compiler decides, based on the static types of the expressions involved.
- 1 What about cases with generics? Like Foo<T>(T a, T b) { a |= b; } – PorkWaffles Commented Nov 28, 2011 at 0:38
- 2 @PorkWaffles: You'd get a compile error, as T is not restricted to an interface/type guaranteed to handle the | operator. "Operator '|=' cannot be applied to operands of type 'T' and 'T'" – Joel B Fant Commented Nov 28, 2011 at 0:40
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c# operators bit-manipulation or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The return of Staging Ground to Stack Overflow
- The 2024 Developer Survey Is Live
Hot Network Questions
- Reviewers do not live up to own quality standards
- Why is “selling a birthright (πρωτοτόκια)” so bad? -- Hebrews 12:16
- Function of R6 in this voltage reference circuit
- Approximation algorithm for binary (linear) programs
- How did the `long` and `short` integer types originate?
- Nonconsecutive Anti-Knight Fillomino?
- Prove that the numbers 2008 and 2106 are not terms of this sequence.
- If "Good luck finding a new job" is sarcastic, how do change the sentence to make it sound well-intentioned?
- Why are amber bottles used to store Water for HPLC?
- How to re-use QGIS map layout for other projects /How to create a print layout template?
- What is the relevance and meaning if a sentence contains "Had not"?
- Will it break Counterspell if the trigger becomes "perceive" instead of "see"?
- Can I enter France on an expired EU passport?
- Are there good examples of regular life being theory-laden?
- Split Flaps and lift?
- Are there any well-known mathematicians who were marxists?
- How to draw a number of circles inscribed in a square so that the sum of the radii of the circle is greatest?
- Is it better to perform multiple paired t-test or One-Way ANOVA test
- Why are heavy metals toxic? Lead and Carbon are in the same group. One is toxic, the other is not
- My supervisor is promoting my work (that I think has flaws) everywhere - what to do?
- Is a judge's completely arbitrary determination of credibilty subject to appeal?
- Hydrogen in Organometallic Chemistry
- What is the difference between "боля" and "болея"?
- LTCGY - Let the corners guide you

IMAGES
VIDEO
COMMENTS
In this article. The assignment operator = assigns the value of its right-hand operand to a variable, a property, or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or ...
C# Assignment Operators Previous Next Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example int x = 10;
Simple assignment operator, Assigns values from right side operands to left side operand. C = A + B assigns value of A + B into C. +=. Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. C += A is equivalent to C = C + A.
The C# specification for compound operators says: 7.17.2 Compound assignment An operation of the form x op= y is processed by applying binary operator overload resolution (§7.3.4) as if the operation was written x op y .
In expressions with the null-conditional operators ?. and ?[], you can use the ?? operator to provide an alternative expression to evaluate in case the result of the expression with null-conditional operations is null:
In this article. C# provides a number of operators. Many of them are supported by the built-in types and allow you to perform basic operations with values of those types. Those operators include the following groups: Arithmetic operators that perform arithmetic operations with numeric operands; Comparison operators that compare numeric operands; Boolean logical operators that perform logical ...
In c#, Assignment Operators are useful to assign a new value to the operand, and these operators will work with only one operand. For example, we can declare and assign a value to the variable using the assignment operator ( =) like as shown below. int a; a = 10; If you observe the above sample, we defined a variable called " a " and ...
The C# Assignment operators are associated with arithmetic operators as a prefix, i.e., +=, -=, *=, /=, %=. The following tables show the available list of C# assignment operators. Symbol Operation Example += Plus Equal To: x+=15 is x=x+15-= Minus Equal To: x- =15 is x=x-15 *= Multiply Equal To: x*=16 is x=x+16 %=
Operators are used to perform various operations on variables and values.. Syntax. The following code snippet uses the assignment operator, =, to set myVariable to the value of num1 and num2 with an arithmetic operator operating on them. For example, if operator represented *, myVariable would be assigned a value of num1 * num2.. myVariable = num1 operator num2;
Assignment Operators. Conditional Operator. In C#, Operators can also categorized based upon Number of Operands : Unary Operator: Operator that takes one operand to perform the operation. Binary Operator: Operator that takes two operands to perform the operation. Ternary Operator: Operator that takes three operands to perform the operation.
The C# assignment operator is generally suffix with arithmetic operators. The symbol of c sharp assignment operator is "=" without quotes. The assignment operator widely used with C# programming. Consider a simple example: result=num1+num2; In this example, the equal to (=) assignment operator assigns the value of num1 + num2 into result ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Assignment operators (Assignment ( =) and compound assignments ( +=, -+, *=, /=, %= )) are used to assign the value or an expression's result to the left side variable, following are the set of assignment operators, "=" - it is used to assign value or an expression's result to the left side variable. "+=" - it is used to add second operand ...
For example, consider the expression 2 + 3 = 5, here 2 and 3 are operands, and + and = are called operators. So, the Operators in C# are used to manipulate the variables and values in a program. int x = 10, y = 20; int result1 = x + y; //Operator Manipulating Variables, where x and y are variables and + is operator.
A user-defined type can overload the + operator. When a binary + operator is overloaded, the += operator is also implicitly overloaded. A user-defined type can't explicitly overload the += operator. C# language specification. For more information, see the Unary plus operator and Addition operator sections of the C# language specification. See also
The copy constructor is the option to go here if you are using classes. A way to use the assignment operator is to use non-referenced types like primitives or a struct. However, a struct should not have functions! That's what classes are for.
Assignment operator. Assignment operator. The Assignment operator is the single equal sign =.it has this general form. Var=expression; Here the type of the var is must compatible with the type of expression.assignment operator has one most important attribute that it allows us to create a chain of assignments. For example.
In SQL, the MINUS operator plays a crucial role in querying by allowing developers to identify and retrieve records that exist in one dataset but not in another. This article explores the functionality, usage, and practical applications of the MINUS operator in SQL, highlighting its significance in data analysis and manipulation tasks.
We have equivalent assignment operators for all Logical operators, Shift operators, Additive operators and all Multiplicative operators. Why did the logical operators get left out? Is there a good technical reason why it is hard? ... Short circuit on |= and &= assignment operators in C#. 5. What is happening when you use the |= operator in C#? 3.
Assignment Operators in Python. Let's see an example of Assignment Operators in Python. Example: The code starts with 'a' and 'b' both having the value 10. It then performs a series of operations: addition, subtraction, multiplication, and a left shift operation on 'b'.
3. No, this is not possible. The assignment operator is not overridable and for good reason. Developers are, even within the confines of C# and modern object-oriented programming languages, often "abusing" the system to make it do things it's not supposed to do.
Operator overloadability. A user-defined type can't overload the conditional operator. C# language specification. For more information, see the Conditional operator section of the C# language specification. Specifications for newer features are: Target-typed conditional expression; See also. Simplify conditional expression (style rule IDE0075)
C# AND assignment operator (&=) with bitwise enums. 0. Bitwise operation in C#. Hot Network Questions Clipping buffers by Unique ID Who was the first philosopher to describe what we now call artificial intelligence? Word for a country declaring independence from an empire Photo on a CV for a researcher application in Japan? ...