Vertex Academy
How to assign a value to a variable in java.

The assigning of a value to a variable is carried out with the help of the "=" symbol. Consider the following examples:

Example No.1
Let’s say we want to assign the value of "10" to the variable "k" of the "int" type. It’s very easy and can be done in two ways:
If you try to run this code on your computer, you will see the following:
In this example, we first declared the variable to be "k" of the "int" type:
Then in another line we assigned the value "10" to the variable "k":
As you you may have understood from the example, the "=" symbol is an assignment operation. It always works from right to left:

This assigns the value "10" to the variable "k."
As you can see, in this example we declared the variable as "k" of the int type and assigned the value to it in a single line:
int k = 10;
So, now you know that:
- The "=" symbol is responsible for assignment operation and we assign values to variables with the help of this symbol.
- There are two ways to assign a value to variables: in one line or in two lines.
What is variable initialization?
Actually, you already know what it is. Initialization is the assignment of an initial value to a variable. In other words, if you just created a variable and didn’t assign any value to it, then this variable is uninitialized. So, if you ever hear:
- “We need to initialize the variable,” it simply means that “we need to assign the initial value to the variable.”
- “The variable has been initialized,” it simply means that “we have assigned the initial value to the variable.”
Here's another example of variable initialization:
Example No.2
15 100 100000000 I love Java M 145.34567 3.14 true
In this line, we declared variable number1 of the byte type and, with the help of the "=" symbol, assigned the value 15 to it.
In this line, we declared variable number2 of the short type and, with the help of the "=" symbol, assigned the value 100 to it.
In this line, we declared variable number3 of the long type and, with the help of the "=" symbol, assigned the value 100000000 to it.
In this line, we declared the variable title of the string type and, with the help of the "=" symbol, assigned the value “I love Java” to it. Since this variable belongs to the string type, we wrote the value of the variable in double quotes .
In this line, we declared the variable letter of the char type and, with the help of the "=" symbol, assigned the value “M” to it. Note that since the variable belongs to the char type, we wrote the value of the variable in single quotes .
In this line, we declared the variable sum of the double type and, with the help of the "=" symbol, assigned the value "145.34567" to it.
In this line, we declared the variable pi of the float type and, with the help of the "=" symbol, assigned the value “3.14f” to it. Note that we added f to the number 3.14. This is a small detail that you'll need to remember: it's necessary to add f to the values of float variables. However, when you see it in the console, it will show up as just 3.14 (without the "f").
In this line, we declared the variable result of the boolean type and, with the help of the "=" symbol, assigned the value "true" to it.
Then we display the values of all the variables in the console with the help of
LET’S SUMMARIZE:
- We assign a value to a variable with the help of the assignment operator "=." It always works from right to left.

2. There are two ways to assign a value to a variable:
- in two lines
- or in one line
- You also need to remember:
If we assign a value to a variable of the string type, we need to put it in double quotes :
If we assign a value to a variable of the char type, we need to put it in single quotes :
If we assign a value to a variable of the float type, we need to add the letter "f" :
- "Initialization" means “to assign an initial value to a variable.”

- ← How do you add comments to your code?
- Operators in Java →
You May Also Like
Software configuration on windows, software configuration, software configuration on ubuntu.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Rules For Variable Declaration in Java
Variable in Java is a data container that saves the data values during Java program execution. Every variable is assigned a data type that designates the type and quantity of value it can hold. Variable is a memory location name of the data. A variable is a name given to a memory location. For More On Variables please check Variables in Java .
Syntax:

Rules to Declare a Variable
- A variable name can consist of Capital letters A-Z , lowercase letters a-z digits 0-9 , and two special characters such as _ underscore and $ dollar sign.
- The first character must not be a digit.
- Blank spaces cannot be used in variable names.
- Java keywords cannot be used as variable names.
- Variable names are case-sensitive.
- There is no limit on the length of a variable name but by convention, it should be between 4 to 15 chars.
- Variable names always should exist on the left-hand side of assignment operators.
List of Java keywords
The table above shows the list of all java keywords that programmers can not use for naming their variables, methods, classes, etc. The keywords const and goto are reserved, but they are not currently used. The words true, false, and null might seem like keywords, but they are actually literals, you cannot use them as identifiers in your programs.
Here are a few valid Java variable name examples:
myvar myVar MYVAR _myVar $myVar myVar1 myVar_1
Similar Reads
- java-basics
Please Login to comment...
Improve your coding skills with practice.
What kind of Experience do you want to share?

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = operator. For example:
In the above assignment statement, the result of x + 1 is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1 ≠ the Java expression x = 2 * x + 1
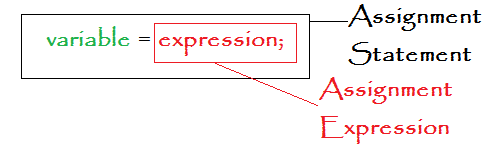
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | ◘
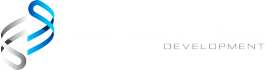
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages


COMMENTS
Variables are containers for storing data values. In Java, there are different typesof variables, for example: 1. String- stores text, such as "Hello". String values are surrounded by double quotes 2. int- st…
In Java, your variables can be split into two categories: Objects, and everything else (int, long, byte, etc). A primitive type (int, long, etc), holds whatever value you assign it. An …
In Java, Variables are the data containers that save the data values during Java program execution. Every Variable in Java is assigned a data type that designates the type and quantity of value it can hold. A variable is a …
Assignment Operators. These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of …
In Java 10, this is how we could declare a local variable: @Test public void whenVarInitWithString_thenGetStringTypeVar() { var message = "Hello, Java 10"; assertTrue(message instanceof String); }
The assigning of a value to a variable is carried out with the help of the "=" symbol. Consider the following examples: Example No.1. Let’s say we want to assign the value of "10" to the variable "k" of the "int" type. It’s very …
Variable in Java is a data container that saves the data values during Java program execution. Every variable is assigned a data type that designates the type and quantity of value it can hold. Variable is a memory …
After a variable is declared, you can assign a value to it by using an assignment statement. In Java, the equal sign = is used as the assignment operator. The syntax for assignment statements is as follows: variable = expression;