- The Ternary Operator in TypeScript
- TypeScript Howtos

TypeScript Operators
Use the ternary operator in typescript, implement nested conditions with the ternary operator in typescript.
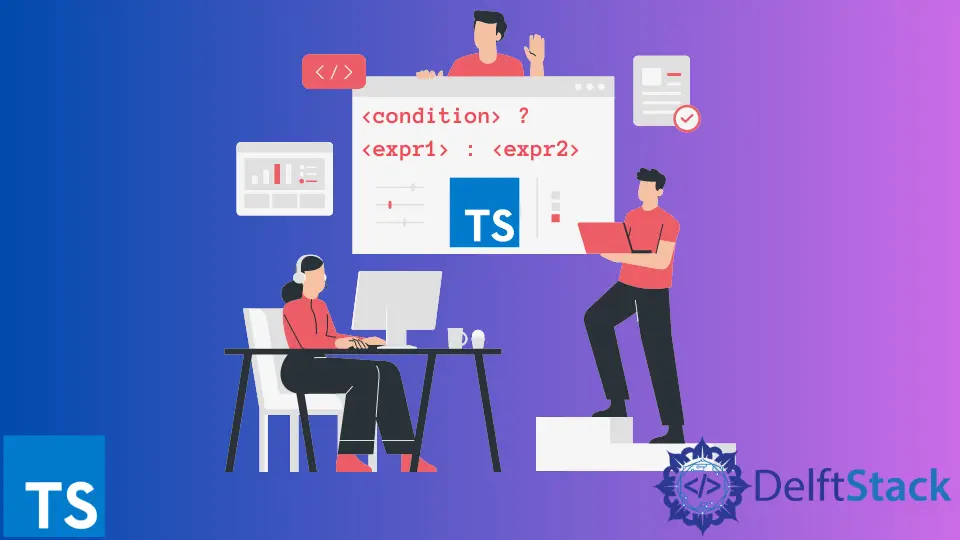
In this article, we will briefly introduce the different operators in TypeScript and discuss the ternary operators and how to use them.
Software applications are intended to work with data. Hence, they have designed a way to perform different operations on this data.
Each operation works with one or more data values and generates a final result. These operations can be divided into various groups.
Operands and Operators in TypeScript
Usually, an operator operates on at least one data value called an operand. For example, in the expression 500 + 200 , the values 500 and 200 are two operands, and the + is the operator.
Several operators can be seen in TypeScript. These can be divided into groups based on the nature of each operator’s operation, such as arithmetic, logical, bitwise, relational, etc.
Also, these operators can be grouped based on the number of operands each operator expects. The binary operator has two operands, as shown in the following.
The unary operator takes only one operand.
TypeScript language supports a ternary operator that operates on three operands; it is the shortened format of the if...else syntax. We call it the TypeScript conditional operator.
The TypeScript conditional operator takes three operands. The first is the condition to be evaluated; it can be identified as the if() part in the usual if...else syntax.
The next two operands are the two expressions to be executed based on the evaluated condition results. Hence, the second operand is the expression to be executed when the condition is evaluated to true .
Otherwise, the third operand expression is returned.
- <your_condition> is the condition to be evaluated. It is a boolean expression that returns either true or false .
- <expression_A> is the expression to be returned when the condition is true .
- <expression_B> is the expression to be returned when the condition is false .
The conditional operator is the only ternary operator available in the TypeScript language.
Let’s write a TypeScript code to check the user’s age, which will return a message based on that. First, we will write the conditional logic using ordinary if...else .
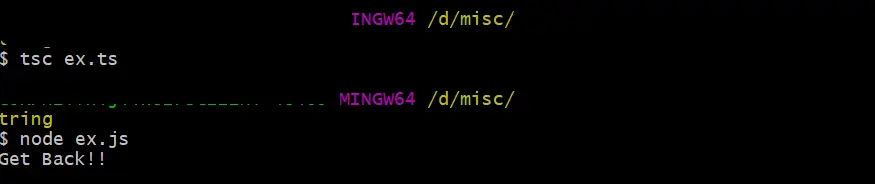
The same logic can be written more compactly using the ternary operator.
You will be getting the same output as in the above if...else logic. This is fewer lines than the if...else syntax and is cleaner.
The ternary operator is not limited to a single condition. It also supports multiple conditions.
Let’s look at the nested if...else conditional logic, as shown in the following.

Let’s write the nested conditions above using the ternary operator.
If you transpile the above TypeScript code and run it using node, you will be getting the same output as in the above if...else scenario.
It is recommended to use a conditional operator in your code. It is a one-line expression that makes your code cleaner.
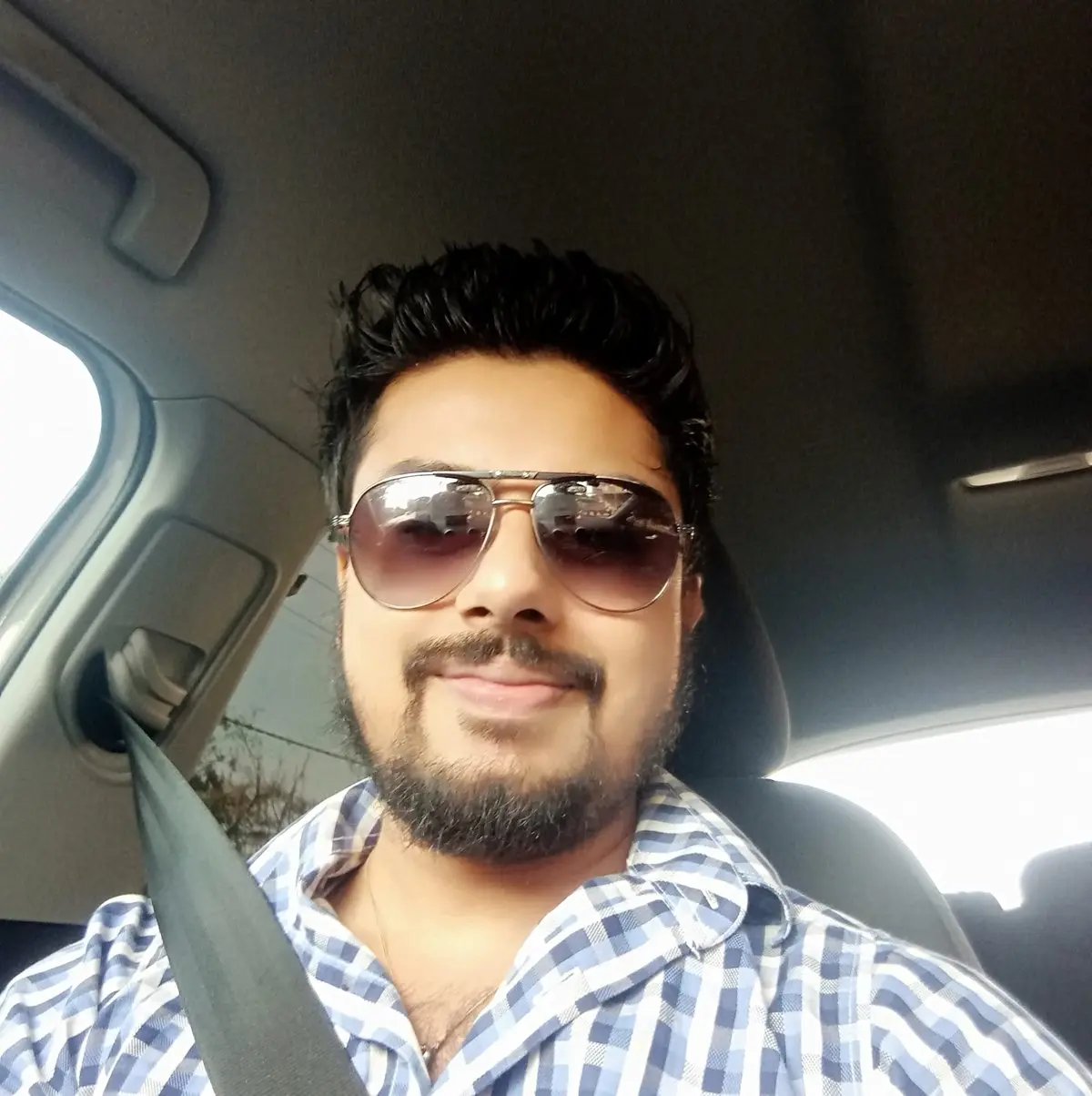
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
Related Article - TypeScript Operator
- TypeScript in Operator
- How to Use the keyof Operator in TypeScript
- Exclamation Mark in TypeScript
- Question Mark Operator in TypeScript
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Staging Ground badges
Earn badges by improving or asking questions in Staging Ground.
Is it (`?:`) typescript ternary operator [duplicate]
I saw the code below in a TypeScript example:
What does ?: mean? Is it a ternary operator (with only false condition) or something else?
- 3 typescriptlang.org/docs/handbook/interfaces.html – J. Steen Commented Apr 12, 2017 at 11:07
- 4 the ? is used for Optional Properties in an interface. typescriptlang.org/docs/handbook/interfaces.html – Claies Commented Apr 12, 2017 at 11:09
- 1 to make fields optional in typescript – Pardeep Jain Commented Apr 12, 2017 at 11:11
- xplatform.rocks/2016/01/07/angular2-quicky-elvis-in-tha-house – Yoav Schniederman Commented Apr 12, 2017 at 11:40
4 Answers 4
The ? operator indicate that the property can be nullable / optional. It just means that the compilator will not throw an error if you do not implement this property in your implementation.

- 1 same as in .NET – mihail Commented Apr 12, 2017 at 11:31
- 1 Except that .NET also has a null-coalescing ?? operator. @mihail – Jnr Commented Jan 31, 2019 at 7:45
- Nullable/optional are not the same thing. Read more here: medium.com/@angela.amarapala/… – Arnab Datta Commented Dec 16, 2019 at 11:02
You can use ?? operator!
This will print 'none' because test is null. If there is a value for test, it will print that. You can try that here playground typescript

- Your code is nullable variable declaration
But the symbol of ?: using from Elvis operator
It Code looks like
And it's not available in typescript/javascript/angular and essentially the same as ||
More details : Comparison of Ternary operator, Elvis operator, safe Navigation Operator and logical OR operators
The Elvis operator is only available for the . not for other dereference operators like [].
As a workaround use
- 1 xplatform.rocks/2016/01/07/angular2-quicky-elvis-in-tha-house – Yoav Schniederman Commented Apr 12, 2017 at 11:40

Not the answer you're looking for? Browse other questions tagged typescript or ask your own question .
- The Overflow Blog
- Rust is evolving from system-level language to UI and frontend development
- Featured on Meta
- Preventing unauthorized automated access to the network
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- Proposed designs to update the homepage for logged-in users
- Feedback Requested: How do you use the tagged questions page?
Hot Network Questions
- What were the 3 charges of "attempting to influence a public servant" in the Tina Peters case for?
- How did “way to go” come to mean “well done”?
- How many different (yet equivalent) definitions of normal subgroups are there?
- Topology Blender
- Can weapon special abilities be activated at the same time with a single command?
- Why does the Fisker Ocean have such a low top speed?
- Why is the foundation of a passive portfolio better than active?
- Conditional Probability: Making the right assumptions
- How to itemise with a loop for a given variable with ; as delimiters
- Applying to two PhD positions under the same professor at the same time?
- Is LetsEncrypt activity Public?
- using cat command with different outputs in a loop
- Meaning of the joke A: "how much coke do you do" B: "Yes."
- Would it be possible for a planet to have only one lifeform?
- Is the Nobel Prize in Physics 2024 statement of merit incorrect?
- Defining a sequence from a list or a table
- SystemVerilog threads execution order
- 64-pin chips in the 1980s
- Are there any special actions that I should execute as a reviewer of a sloppy manuscript?
- PostgreSQL unaccent and full text search for Arabic/Persian
- Decide symmetry of fractions
- What kind of green-leafed flower is this?
- Why "geometria" and not "geometrica"?
- Why do apps such as the DuckDuckGo browser or Signal seem to try to protect my own data from me?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
- Português (do Brasil)
Conditional (ternary) operator
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark ( ? ), then an expression to execute if the condition is truthy followed by a colon ( : ), and finally the expression to execute if the condition is falsy . This operator is frequently used as an alternative to an if...else statement.
An expression whose value is used as a condition.
An expression which is executed if the condition evaluates to a truthy value (one which equals or can be converted to true ).
An expression which is executed if the condition is falsy (that is, has a value which can be converted to false ).
Description
Besides false , possible falsy expressions are: null , NaN , 0 , the empty string ( "" ), and undefined . If condition is any of these, the result of the conditional expression will be the result of executing the expression exprIfFalse .
A simple example
Handling null values.
One common usage is to handle a value that may be null :
Conditional chains
The ternary operator is right-associative, which means it can be "chained" in the following way, similar to an if … else if … else if … else chain:
This is equivalent to the following if...else chain.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Nullish coalescing operator ( ?? )
- Optional chaining ( ?. )
- Making decisions in your code — conditionals
- Expressions and operators guide
