- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
- Português (do Brasil)

Baseline Widely available
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The while statement creates a loop that executes a specified statement as long as the test condition evaluates to true. The condition is evaluated before executing the statement.
An expression evaluated before each pass through the loop. If this condition evaluates to true , statement is executed. When condition evaluates to false , execution continues with the statement after the while loop.
A statement that is executed as long as the condition evaluates to true. You can use a block statement to execute multiple statements.
Description
Like other looping statements, you can use control flow statements inside statement :
- break stops statement execution and goes to the first statement after the loop.
- continue stops statement execution and re-evaluates condition .
Using while
The following while loop iterates as long as n is less than three.
Each iteration, the loop increments n and adds it to x . Therefore, x and n take on the following values:
- After the first pass: n = 1 and x = 1
- After the second pass: n = 2 and x = 3
- After the third pass: n = 3 and x = 6
After completing the third pass, the condition n < 3 is no longer true, so the loop terminates.
Using an assignment as a condition
In some cases, it can make sense to use an assignment as a condition. This comes with readability tradeoffs, so there are certain stylistic recommendations that would make the pattern more obvious for everyone.
Consider the following example, which iterates over a document's comments, logging them to the console.
That's not completely a good-practice example, due to the following line specifically:
The effect of that line is fine — in that, each time a comment node is found:
- iterator.nextNode() returns that comment node, which gets assigned to currentNode .
- The value of currentNode = iterator.nextNode() is therefore truthy .
- So the console.log() call executes and the loop continues.
…and then, when there are no more comment nodes in the document:
- iterator.nextNode() returns null .
- The value of currentNode = iterator.nextNode() is therefore also null , which is falsy .
- So the loop ends.
The problem with this line is: conditions typically use comparison operators such as === , but the = in that line isn't a comparison operator — instead, it's an assignment operator . So that = looks like it's a typo for === — even though it's not actually a typo.
Therefore, in cases like that one, some code-linting tools such as ESLint's no-cond-assign rule — in order to help you catch a possible typo so that you can fix it — will report a warning such as the following:
Expected a conditional expression and instead saw an assignment.
Many style guides recommend more explicitly indicating the intention for the condition to be an assignment. You can do that minimally by putting additional parentheses as a grouping operator around the assignment:
In fact, this is the style enforced by ESLint's no-cond-assign 's default configuration, as well as Prettier , so you'll likely see this pattern a lot in the wild.
Some people may further recommend adding a comparison operator to turn the condition into an explicit comparison:
There are other ways to write this pattern, such as:
Or, forgoing the idea of using a while loop altogether:
If the reader is sufficiently familiar with the assignment as condition pattern, all these variations should have equivalent readability. Otherwise, the last form is probably the most readable, albeit the most verbose.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
Home » JavaScript Tutorial » JavaScript while Loop
JavaScript while Loop
Summary : in this tutorial, you will learn how to use the JavaScript while statement to create a loop that executes a block as long as a condition is true .
Introduction to the JavaScript while loop statement
The JavaScript while statement creates a loop that executes a block as long as a condition evaluates to true .
The following illustrates the syntax of the while statement:
The while statement evaluates the expression before each iteration of the loop.
If the expression evaluates to true , the while statement executes the statement . Otherwise, the while loop exits.
Because the while loop evaluates the expression before each iteration, it is known as a pretest loop.
If the expression evaluates to false before the loop enters, the while loop will never execute.
The following flowchart illustrates the while loop statement:
Note that if you want to execute the statement a least once and check the condition after each iteration, you should use the do…while statement.
JavaScript while loop examples
Let’s take some examples of using the JavaScript while loop statement.
Basic JavaScript while loop example
The following example uses the while statement to output the odd numbers between 1 and 10 to the console:
How the script works
First, declare and initialize the count variable to 1 :
Second, execute the statement inside the loop if the count variable is less than 10 . In each iteration, output the count to the console and increase the count by 2 :
Third, after 5 iterations, the count is 11 . Therefore, the condition count < 10 is false , the loop exits.
Calculating the sum of a sequence of numbers
The following example uses the while loop to calculate the sum of sequence of numbers from 1 to 100:
How it works.
First, declare and initialize some variables:
- The total variable stores the sum of numbers between 1 and 100 .
- The n variable serves as the ending number.
- The i variable is a loop variable.
Second, add value of i to the total as long as i is less than or equal to 100 :
Note that you need to increment i by one in each iteration. If you don’t do so, the condition i <= 100 will always true , which causes an indefinite loop.
Third, display the total to the console:
Using while loop with an array
The following example uses the while loop to iterate over elements of an array :
First, define an array that stores mountain names:
Second, declare and initialize a loop variable ( i ) to zero:
Third, run the loop as long as the loop variable ( i ) is less than the number of elements in the mountains array:
Finally, show the mountain names and increment the loop variable ( i ) in each iteration:
Since the mountains array has four elements, the while loop performs four iterations, each per element.
- Use a while loop statement to create a loop that executes a block as long as a condition is true .
Popular Tutorials
Popular examples, reference materials, certification courses.
Created with over a decade of experience and thousands of feedback.
JS Introduction
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
JavaScript for loop
- JavaScript while loop
JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript for... of Loop
JavaScript Ternary Operator
JavaScript while and do...while Loop
- JavaScript while Loop
The while loop repeatedly executes a block of code as long as a specified condition is true .
The syntax of the while loop is:
- The while loop first evaluates the condition inside ( ) .
- If the condition evaluates to true , the code inside { } is executed.
- Then, the condition is evaluated again.
- This process continues as long as the condition evaluates to true .
- If the condition evaluates to false , the loop stops.
Flowchart of while Loop
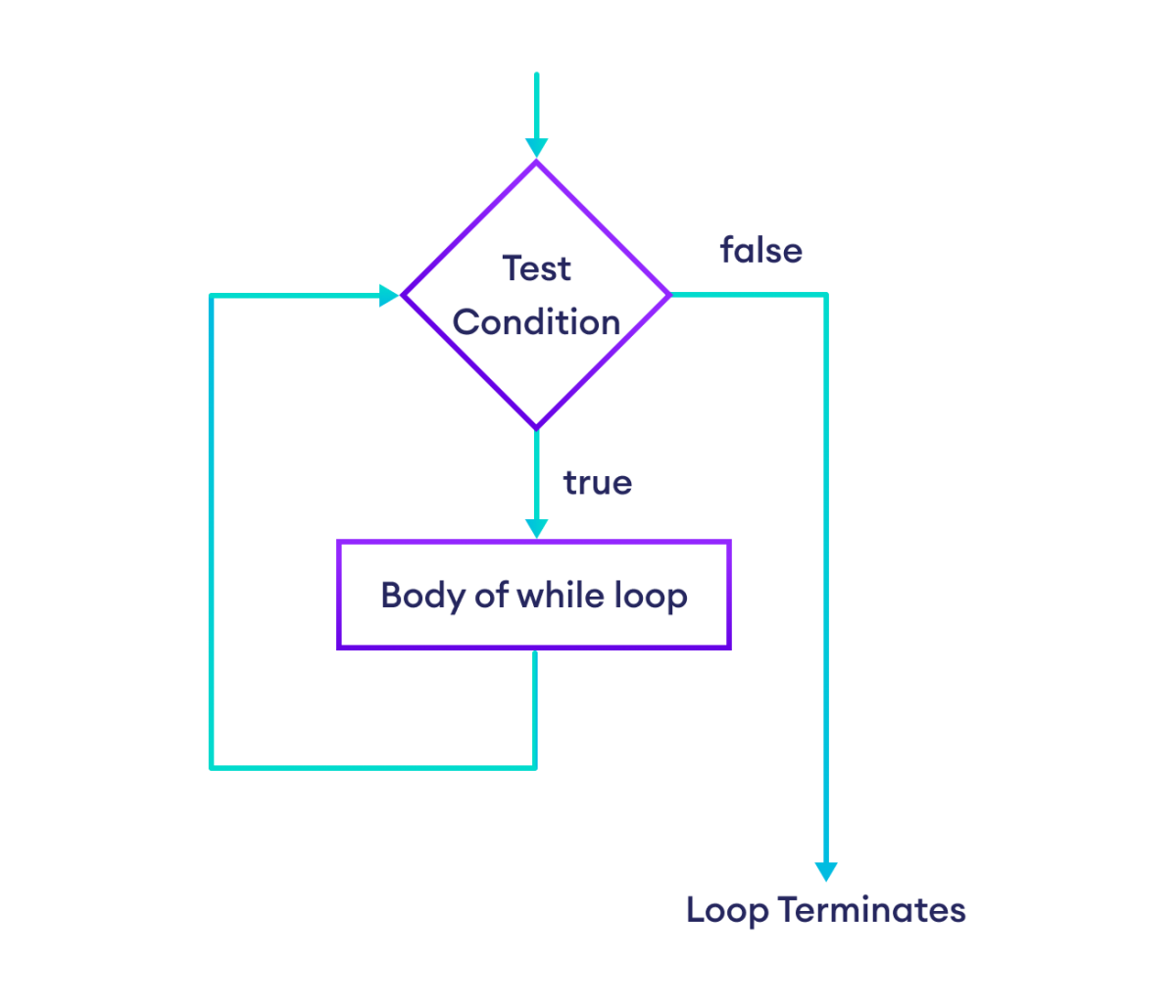
- Example 1: Display Numbers From 1 to 3
Here is how the above program works in each iteration of the loop:
To learn more about loop conditions, visit JavaScript Comparison and Logical Operators .
- Example 2: Sum of Only Positive Numbers
The above program prompts the user to enter a number.
Since JavaScript prompt() only takes inputs as string , parseInt() converts the input to a number.
As long as we enter positive numbers, the while loop adds them up and prompts us to enter more numbers.
So when we enter a negative number, the loop terminates.
Finally, we display the total sum of positive numbers.
Note: When we add two or more numeric strings, JavaScript treats them as strings. For example, "2" + "3" = "23" . So, we should always convert numeric strings to numbers to avoid unexpected behaviors.
- JavaScript do...while Loop
The do...while loop executes a block of code once, then repeatedly executes it as long as the specified condition is true .
The syntax of the do...while loop is:
- The do…while loop executes the code inside { } .
- Then, it evaluates the condition inside ( ) .
- If the condition evaluates to true , the code inside { } is executed again.
- If the condition evaluates to false , the loop terminates.
Flowchart of do...while Loop
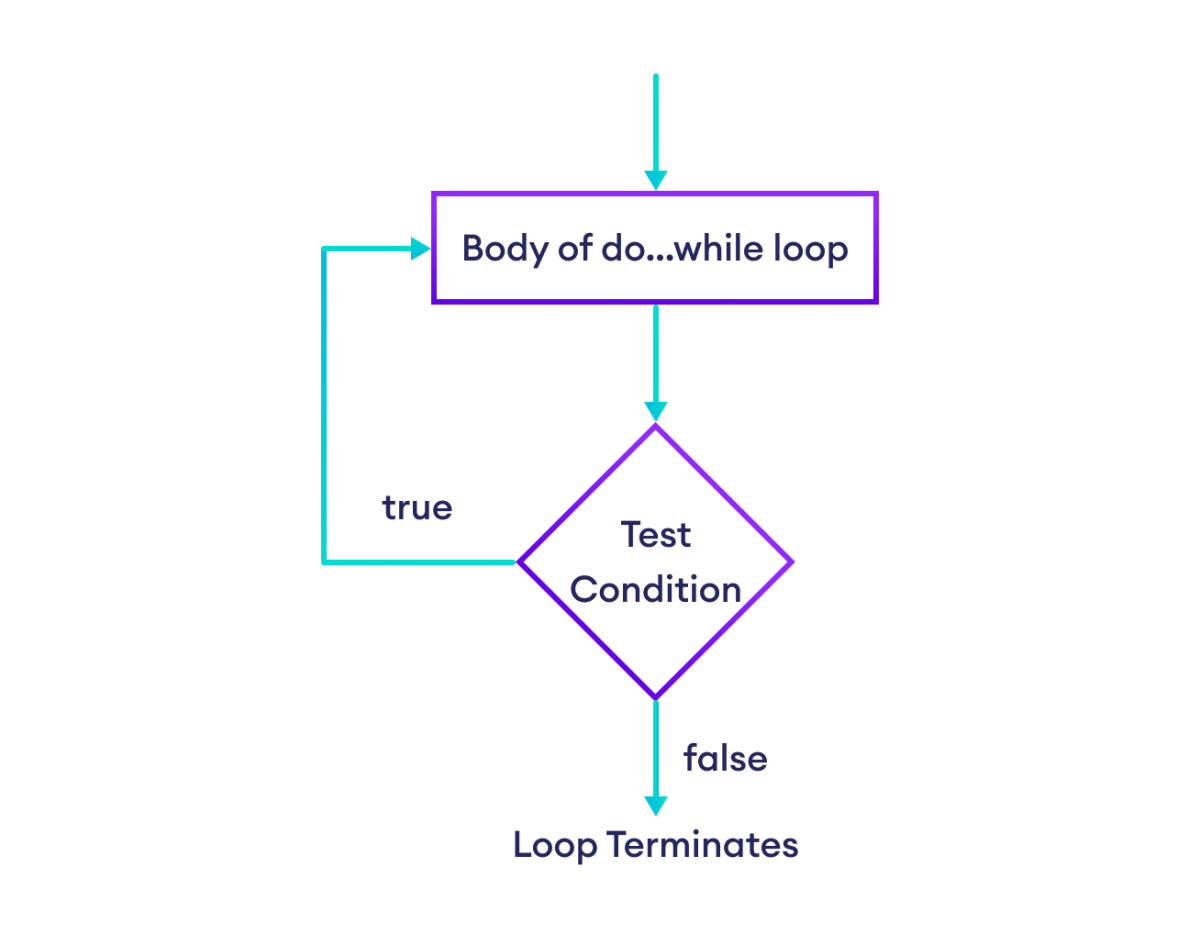
- Example 3: Display Numbers from 3 to 1
Here, the initial value of i is 3 . Then, we used a do...while loop to iterate over the values of i . Here is how the loop works in each iteration:
The difference between while and do...while is that the do...while loop executes its body at least once. For example,
On the other hand, the while loop doesn't execute its body if the loop condition is false . For example,
- Example 4: Sum of Positive Numbers
In the above program, the do...while loop prompts the user to enter a number.
As long as we enter positive numbers, the loop adds them up and prompts us to enter more numbers.
If we enter a negative number, the loop terminates without adding the negative number.
More on JavaScript while and do...while Loops
An infinite while loop is a condition where the loop runs infinitely, as its condition is always true . For example,
Also, here is an example of an infinite do...while loop,
In the above program, the condition 1 > 1 is always true , which causes the loop body to run forever.
Note: Infinite loops can cause your program to hang. So, avoid creating them unintentionally.
We use a for loop when we need to perform a fixed number of iterations. For example,
Meanwhile, we use a while loop when the termination condition can vary. For example,
In the above program, we let the user print hi as much as they desire.
Since we don't know the user's decision, we use a while loop instead of a for loop.
Table of Contents
Before we wrap up, let’s put your knowledge of JavaScript while and do...while Loop to the test! Can you solve the following challenge?
Write a function to calculate the factorial of a number.
- The factorial of a non-negative integer n is the product of all positive integers less than or equal to n .
- For example, the factorial of 3 is 3 * 2 * 1 = 6 .
- Return the factorial of the input number num .
Video: JavaScript while Loop
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
JavaScript Tutorial

JavaScript While Loop
In this tutorial, you will learn about the while loop, how to use it in JavaScript with the flow diagram and examples.
While loop is a control flow statement that is used to execute a block of code over and over again until the condition given is true.
Condition is evaluated before execution enters the loop body. If the condition is true, the loop body is executed. If the condition is false, the loop body is skipped and the loop continues.
while loop is also known as pre-test loop because it executes statement before execution of body.
While loop syntax
The syntax of the while loop is as follows:
- The while keyword is followed by a condition.
- The condition is enclosed in parenthesis.
- A condition can be anything boolean, number, expression, etc, but must be evaluated to true or false.
- The body is enclosed in curly braces.
Flow diagram
The flow diagram below shows the execution of the while loop.

while loop javascript example
Here are some examples of while loop in JavaScript.
Code Explanation:
- First, the variable count is initialized to 0
- Then, before entering the loop condition is checked
- If the condition is true, the loop body is executed
- After executing the loop body, the variable count is incremented by 1
- The loop condition is checked again and the loop body is executed again and again until the condition is false
In the above example condition given is just count , which is valid because it is a number and the boolean value of all positive and negative numbers is true.
Loop breaks when count == 0 because boolean value of 0 is false.
When to use while loop
While loop is best suited to be used when you do not know the number of iterations need to reach the result and the only condition is known.
For example, print pages until the paper in the machine are finished. In this case, you don't know how many pages are printed and you only know to keep printing until the paper is finished.
Code for this will look like this:
Let's see few examples:
Example 1: Add numbers of array from start until sum >= 100
Nested while loop
You can also use while loop inside another while loop, this is called nested while loop .
Using Variable in while loop
In while loop, the variable can be used to store the value of a condition.
The count variable in the above example can be used in the loop body to get desired output like executing equations.
Let's see an example to find the sum of even numbers from 1 to 100.
Do while loop
The do-while loop is similar to the while loop, but in the do-while loop, the condition is checked before the body is executed.
It is used only you have a condition to satisfy and you want to execute the loop body atleast once.
The syntax of do-while loop is as follows:
The do keyword is followed by a body enclosed in curly braces and a condition is enclosed in parenthesis.
The following example shows the execution of the do-while loop.
Difference between for and while loop in javascript
There are the following differences between for and while loop in javascript:
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.

Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript While Loop
The while loop executes a block of code as long as a specified condition is true. In JavaScript, this loop evaluates the condition before each iteration and continues running as long as the condition remains true. The loop terminates when the condition becomes false, enabling dynamic and repeated operations based on changing conditions.
Example: Here’s an example of a while loop that counts from 1 to 5.
Do-While loop
A Do-While loop is another type of loop in JavaScript that is similar to the while loop, but with one key difference: the do-while loop guarantees that the block of code inside the loop will be executed at least once, regardless of whether the condition is initially true or false .
Example : Here’s an example of a do-while loop that counts from 1 to 5.
Comparison between the while and do-while loop:
The do-while loop executes the content of the loop once before checking the condition of the while loop. While the while loop will check the condition first before executing the content.
JavaScript While Loop – FAQs
What is a while loop in javascript.
A while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The loop continues to execute as long as the specified condition evaluates to true.
What is the basic syntax of a while loop?
The basic syntax of a while loop includes the while keyword followed by a condition in parentheses and the code block to be executed in curly braces.
How does the condition part work?
The condition part is evaluated before each iteration of the loop. If the condition evaluates to true, the loop body is executed. If it evaluates to false, the loop terminates.
What happens if the condition is always true?
If the condition is always true, the loop will run indefinitely, creating an infinite loop. This can crash your program or browser.
How can you break out of a while loop early?
You can use the break statement to exit a while loop before it completes all its iterations. This is useful if you want to stop the loop based on a condition inside the loop body.
How can you skip an iteration in a while loop?
You can use the continue statement to skip the current iteration and proceed to the next iteration of the loop. This is useful if you want to skip certain iterations based on a condition.
Can you nest while loops in JavaScript?
Yes, you can nest while loops, meaning you can place one while loop inside another while loop. This allows for more complex iterations and conditions.
Similar Reads
- Web Technologies
- javascript-basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
COMMENTS
The while statement creates a loop that executes a specified statement as long as the test condition evaluates to true. The condition is evaluated before executing the statement.
A pure assignment (without instantiation) is coerced to a boolean via its default return value (the value of the right-hand-side). A var (or let or const ) is a statement that allows an optional assignment but has a return value of undefined .
Description. The while statement creates a loop (araund a code block) that is executed while a condition is true. The loop runs while the condition is true. Otherwise it stops. See Also: The JavaScript while Tutorial. JavaScript Loop Statements. Syntax. while (condition) { code block to be executed. } Parameters. Note.
The While Loop. The while loop loops through a block of code as long as a specified condition is true. Syntax
The JavaScript while statement creates a loop that executes a block as long as a condition evaluates to true. The following illustrates the syntax of the while statement: while (expression) { // statement} Code language: JavaScript (javascript) The while statement evaluates the expression before each iteration of the loop. If the expression ...
The JavaScript while and do…while loops repeatedly execute a block of code as long as a specified condition is true. In this tutorial, you will learn about the JavaScript while and do…while loops with examples.
While loop is a control flow statement that is used to execute a block of code over and over again until the condition given is true. Condition is evaluated before execution enters the loop body. If the condition is true, the loop body is executed.
This article explains why I have a warning if I use a code like this: var htmlCollection = document.getElementsByClassName("class-name"), i = htmlCollection.length, htmlElement; // Because htmlCollection is Live, we use a reverse iteration. while (htmlElement = htmlCollection[--i]) { // **Warning?!
The Logical AND assignment operator is used between two values. If the first value is true, the second value is assigned.
What is a while loop in JavaScript? A while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The loop continues to execute as long as the specified condition evaluates to true.