Conditional branching: if, '?'
Sometimes, we need to perform different actions based on different conditions.
To do that, we can use the if statement and the conditional operator ? , that’s also called a “question mark” operator.

The “if” statement
The if(...) statement evaluates a condition in parentheses and, if the result is true , executes a block of code.
For example:
In the example above, the condition is a simple equality check ( year == 2015 ), but it can be much more complex.
If we want to execute more than one statement, we have to wrap our code block inside curly braces:
We recommend wrapping your code block with curly braces {} every time you use an if statement, even if there is only one statement to execute. Doing so improves readability.
Boolean conversion
The if (…) statement evaluates the expression in its parentheses and converts the result to a boolean.
Let’s recall the conversion rules from the chapter Type Conversions :
- A number 0 , an empty string "" , null , undefined , and NaN all become false . Because of that they are called “falsy” values.
- Other values become true , so they are called “truthy”.
So, the code under this condition would never execute:
…and inside this condition – it always will:
We can also pass a pre-evaluated boolean value to if , like this:
The “else” clause
The if statement may contain an optional else block. It executes when the condition is falsy.
Several conditions: “else if”
Sometimes, we’d like to test several variants of a condition. The else if clause lets us do that.
In the code above, JavaScript first checks year < 2015 . If that is falsy, it goes to the next condition year > 2015 . If that is also falsy, it shows the last alert .
There can be more else if blocks. The final else is optional.
Conditional operator ‘?’
Sometimes, we need to assign a variable depending on a condition.
For instance:
The so-called “conditional” or “question mark” operator lets us do that in a shorter and simpler way.
The operator is represented by a question mark ? . Sometimes it’s called “ternary”, because the operator has three operands. It is actually the one and only operator in JavaScript which has that many.
The syntax is:
The condition is evaluated: if it’s truthy then value1 is returned, otherwise – value2 .
Technically, we can omit the parentheses around age > 18 . The question mark operator has a low precedence, so it executes after the comparison > .
This example will do the same thing as the previous one:
But parentheses make the code more readable, so we recommend using them.
In the example above, you can avoid using the question mark operator because the comparison itself returns true/false :
Multiple ‘?’
A sequence of question mark operators ? can return a value that depends on more than one condition.
It may be difficult at first to grasp what’s going on. But after a closer look, we can see that it’s just an ordinary sequence of tests:
- The first question mark checks whether age < 3 .
- If true – it returns 'Hi, baby!' . Otherwise, it continues to the expression after the colon “:”, checking age < 18 .
- If that’s true – it returns 'Hello!' . Otherwise, it continues to the expression after the next colon “:”, checking age < 100 .
- If that’s true – it returns 'Greetings!' . Otherwise, it continues to the expression after the last colon “:”, returning 'What an unusual age!' .
Here’s how this looks using if..else :
Non-traditional use of ‘?’
Sometimes the question mark ? is used as a replacement for if :
Depending on the condition company == 'Netscape' , either the first or the second expression after the ? gets executed and shows an alert.
We don’t assign a result to a variable here. Instead, we execute different code depending on the condition.
It’s not recommended to use the question mark operator in this way.
The notation is shorter than the equivalent if statement, which appeals to some programmers. But it is less readable.
Here is the same code using if for comparison:
Our eyes scan the code vertically. Code blocks which span several lines are easier to understand than a long, horizontal instruction set.
The purpose of the question mark operator ? is to return one value or another depending on its condition. Please use it for exactly that. Use if when you need to execute different branches of code.
if (a string with zero)
Will alert be shown?
Yes, it will.
Any string except an empty one (and "0" is not empty) becomes true in the logical context.
We can run and check:
The name of JavaScript
Using the if..else construct, write the code which asks: ‘What is the “official” name of JavaScript?’
If the visitor enters “ECMAScript”, then output “Right!”, otherwise – output: “You don’t know? ECMAScript!”
Demo in new window
Show the sign
Using if..else , write the code which gets a number via prompt and then shows in alert :
- 1 , if the value is greater than zero,
- -1 , if less than zero,
- 0 , if equals zero.
In this task we assume that the input is always a number.
Rewrite 'if' into '?'
Rewrite this if using the conditional operator '?' :
Rewrite 'if..else' into '?'
Rewrite if..else using multiple ternary operators '?' .
For readability, it’s recommended to split the code into multiple lines.
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
How to Use the Ternary Operator in JavaScript – JS Conditional Example
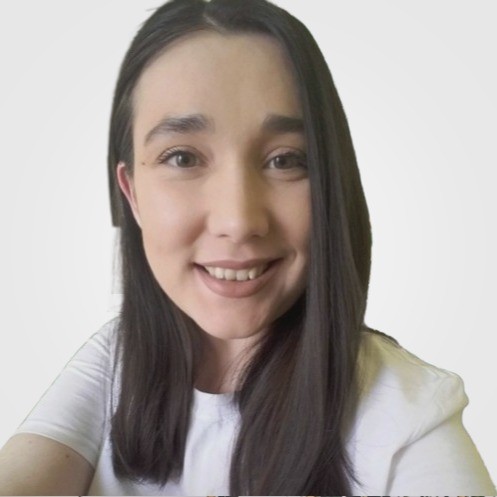
The ternary operator is a helpful feature in JavaScript that allows you to write concise and readable expressions that perform conditional operations on only one line.
In this article, you will learn why you may want to use the ternary operator, and you will see an example of how to use it. You will also learn some of the best practices to keep in mind when you do use it.
Let's get into it!
What Is The Ternary Operator in JavaScript?
The ternary operator ( ?: ), also known as the conditional operator, is a shorthand way of writing conditional statements in JavaScript – you can use a ternary operator instead of an if..else statement.
A ternary operator evaluates a given condition, also known as a Boolean expression, and returns a result that depends on whether that condition evaluates to true or false .
Why Use the Ternary Operator in JavaScript?
You may want to use the ternary operator for a few reasons:
- Your code will be more concise : The ternary operator has minimal syntax. You will write short conditional statements and fewer lines of code, which makes your code easier to read and understand.
- Your code will be more readable : When writing simple conditions, the ternary operator makes your code easier to understand in comparison to an if..else statement.
- Your code will be more organized : The ternary operator will make your code more organized and easier to maintain. This comes in handy when writing multiple conditional statements. The ternary operator will reduce the amount of nesting that occurs when using if..else statements.
- It provides flexibility : The ternary operator has many use cases, some of which include: assigning a value to a variable, rendering dynamic content on a web page, handling function arguments, validating data and handling errors, and creating complex expressions.
- It enhances performance : In some cases, the ternary operator can perform better than an if..else statement because the ternary operator gets evaluated in a single step.
- It always returns a value : The ternary operator always has to return something.
How to Use the Ternary Operator in JavaScript – a Syntax Overview
The operator is called "ternary" because it is composed of three parts: one condition and two expressions.
The general syntax for the ternary operator looks something similar to the following:
Let's break it down:
- condition is the Boolean expression you want to evaluate and determine whether it is true or false . The condition is followed by a question mark, ? .
- ifTrueExpression is executed if the condition evaluates to true .
- ifFalseExpression is executed if the condition evaluates to false .
- The two expressions are separated by a colon, . .
The ternary operator always returns a value that you assign to a variable:
Next, let's look at an example of how the ternary operator works.
How to Use the Ternary Operator in JavaScript
Say that you want to check whether a user is an adult:
In this example, I used the ternary operator to determine whether a user's age is greater than or equal to 18 .
Firstly, I used the prompt() built-in JavaScript function.
This function opens a dialog box with the message What is your age? and the user can enter a value.
I store the user's input in the age variable.
Next, the condition ( age >= 18 ) gets evaluated.
If the condition is true , the first expression, You are an adult , gets executed.
Say the user enters the value 18 .
The condition age >= 18 evaluates to true :
If the condition is false , the second expression, You are not an adult yet , gets executed.
Say the user enters the value 17 .
The condition age >= 18 now evaluates to false :
As mentioned earlier, you can use the ternary operator instead of an if..else statement.
Here is how you would write the same code used in the example above using an if..else statement:
Ternary Operator Best Practices in JavaScript
Something to keep in mind when using the ternary operator is to keep it simple and don't overcomplicate it.
The main goal is for your code to be readable and easily understandable for the rest of the developers on your team.
So, consider using the ternary operator for simple statements and as a concise alternative to if..else statements that can be written in one line.
If you do too much, it can quickly become unreadable.
For example, in some cases, using nested ternary operators can make your code hard to read:
If you find yourself nesting too many ternary operators, consider using if...else statements instead.
Wrapping Up
Overall, the ternary operator is a useful feature in JavaScript as it helps make your code more readable and concise.
Use it when a conditional statement can be written on only one line and keep code readability in mind.
Thanks for reading, and happy coding! :)
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Ternary Operator
JavaScript switch...case Statement
- JavaScript try...catch...finally Statement
- JavaScript if...else Statement
The JavaScript if...else statement is used to execute/skip a block of code based on a condition.
Here's a quick example of the if...else statement. You can read the rest of the tutorial if you want to learn about if...else in greater detail.
In the above example, the program displays You passed the examination. if the score variable is equal to 50 . Otherwise, it displays You failed the examination.
In computer programming, the if...else statement is a conditional statement that executes a block of code only when a specific condition is met. For example,
Suppose we need to assign different grades to students based on their scores.
- If a student scores above 90 , assign grade A .
- If a student scores above 75 , assign grade B .
- If a student scores above 65 , assign grade C .
These conditional tasks can be achieved using the if...else statement.
- JavaScript if Statement
We use the if keyword to execute code based on some specific condition.
The syntax of if statement is:
The if keyword checks the condition inside the parentheses () .
- If the condition is evaluated to true , the code inside { } is executed.
- If the condition is evaluated to false , the code inside { } is skipped.
Note: The code inside { } is also called the body of the if statement.
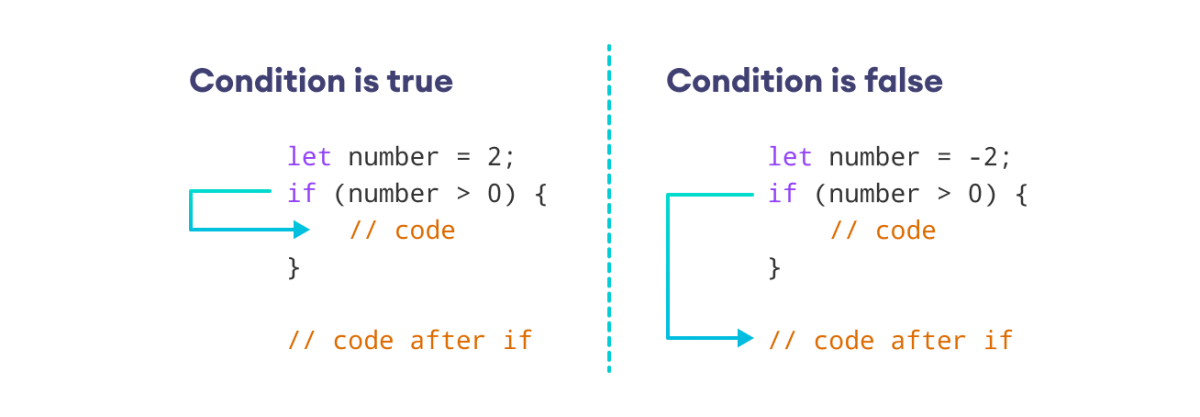
Example 1: JavaScript if Statement
Sample Output 1
In the above program, when we enter 5 , the condition number > 0 evaluates to true . Thus, the body of the if statement is executed.
Sample Output 2
Again, when we enter -1 , the condition number > 0 evaluates to false . Hence, the body of the if statement is skipped.
Since console.log("nice number"); is outside the body of the if statement, it is always executed.
Note: We use comparison and logical operators in our if conditions. To learn more, you can visit JavaScript Comparison and Logical Operators .
- JavaScript else Statement
We use the else keyword to execute code when the condition specified in the preceding if statement evaluates to false .
The syntax of the else statement is:
The if...else statement checks the condition and executes code in two ways:
- If condition is true , the code inside if is executed. And, the code inside else is skipped.
- If condition is false , the code inside if is skipped. Instead, the code inside else is executed.
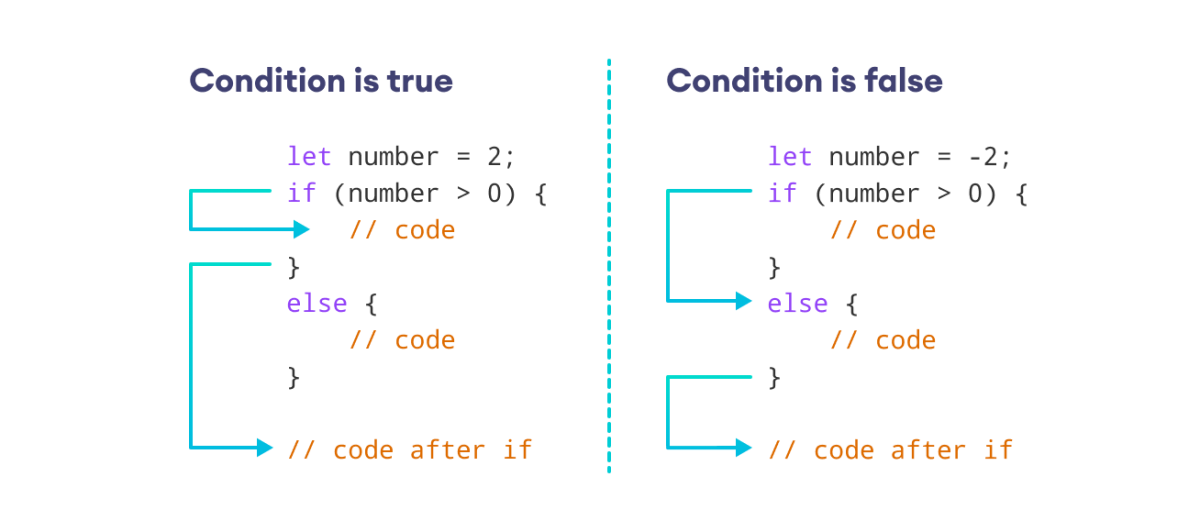
Example 2: JavaScript if…else Statement
In the above example, the if statement checks for the condition age >= 18 .
Since we set the value of age to 17 , the condition evaluates to false .
Thus, the code inside if is skipped. And, code inside else is executed.
We can omit { } in if…else statements when we have a single line of code to execute. For example,
- JavaScript else if Statement
We can use the else if keyword to check for multiple conditions.
The syntax of the else if statement is:
- First, the condition in the if statement is checked. If the condition evaluates to true , the body of if is executed, and the rest is skipped.
- Otherwise, the condition in the else if statement is checked. If true , its body is executed and the rest is skipped.
- Finally, if no condition matches, the block of code in else is executed.
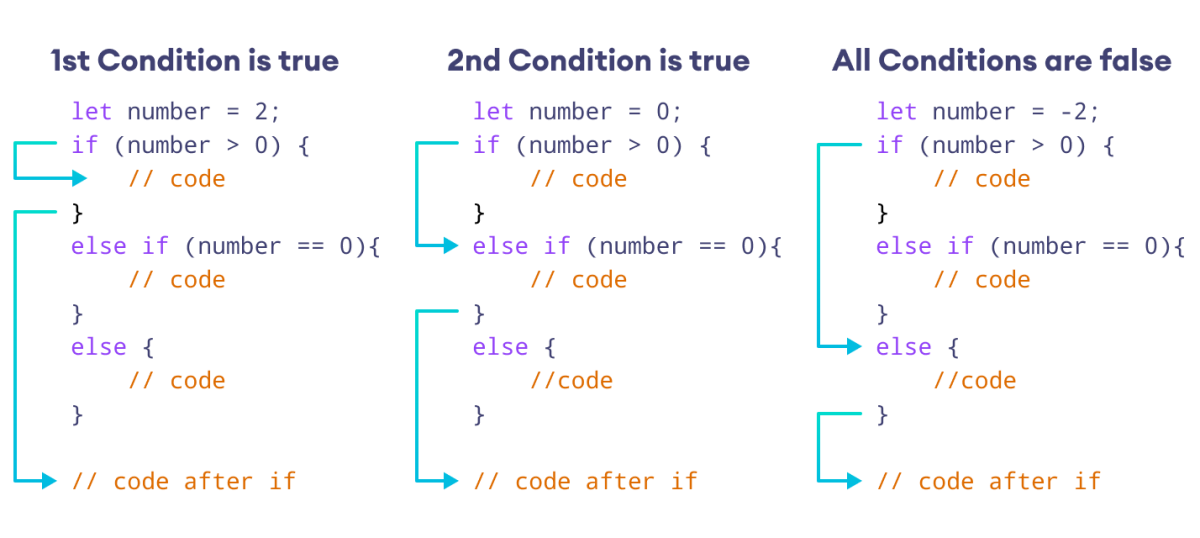
Example 3: JavaScript if...else if Statement
In the above example, we used the if statement to check for the condition rating <= 2 .
Likewise, we used the else if statement to check for another condition, rating >= 4 .
Since the else if condition is satisfied, the code inside it is executed.
We can use the else if keyword as many times as we want. For example,
In the above example, we used two else if statements.
The second else if statement was executed as its condition was satisfied.
- Nested if...else Statement
When we use an if...else statement inside another if...else statement, we create a nested if...else statement. For example,
Outer if...else
In the above example, the outer if condition checks if a student has passed or failed using the condition marks >= 40 . If it evaluates to false , the outer else statement will print Failed .
On the other hand, if marks >= 40 evaluates to true , the program moves to the inner if...else statement.
Inner if...else statement
The inner if condition checks whether the student has passed with distinction using the condition marks >= 80 .
If marks >= 80 evaluates to true , the inner if statement will print Distinction .
Otherwise, the inner else statement will print Passed .
Note: Avoid nesting multiple if…else statements within each other to maintain code readability and simplify debugging.
More on JavaScript if...else Statement
We can use the ternary operator ?: instead of an if...else statement if the operation we're performing is very simple. For example,
can be written as
We can replace our if…else statement with the switch statement when we deal with a large number of conditions.
For example,
In the above example, we used if…else to evaluate five conditions, including the else block.
Now, let's use the switch statement for the same purpose.
As you can see, the switch statement makes our code more readable and maintainable.
In addition, switch is faster than long chains of if…else statements.
We can use logical operators such as && and || within an if statement to add multiple conditions. For example,
Here, we used the logical operator && to add two conditions in the if statement.
Table of Contents
- Introduction
Before we wrap up, let’s put your knowledge of JavaScript if...else Statement to the test! Can you solve the following challenge?
Write a function to check if a student has passed or failed.
- Suppose, the pass mark is 40 .
- If the mark is greater than or equal to 40 , return "Pass" . Otherwise, return "Fail" .
Video: JavaScript if...else
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
JavaScript Tutorial
The JavaScript Ternary Operator as a Shortcut for If/Else Statements
Stone/Cavan Images/Getty Images
- Javascript Programming
- PHP Programming
- Java Programming
- Delphi Programming
- C & C++ Programming
- Ruby Programming
- Visual Basic
- B.S., Computer Science, North Carolina State University
The conditional ternary operator in JavaScript assigns a value to a variable based on some condition and is the only JavaScript operator that takes three operands.
The ternary operator is a substitute for an if statement in which both the if and else clauses assign different values to the same field, like so:
The ternary operator shortens this if/else statement into a single statement:
If condition is true, the ternary operator returns the value of the first expression; otherwise, it returns the value of the second expression. Let's consider its parts:
- First, create the variable to which you want to assign a value, in this case, result . The variable result will have a different value depending on the condition.
- Note that on the right-hand side (i.e. the operator itself), the condition is first.
- The condition is always followed by a question mark ( ? ), which can basically be read as "was that true?"
- The two possible results come last, separated by a colon ( : ).
This use of the ternary operator is available only when the original if statement follows the format shown above — but this is quite a common scenario, and using the ternary operator can be far more efficient.
Ternary Operator Example
Let's look at a real example.
Perhaps you need to determine which children are the right age to attend kindergarten. You might have a conditional statement like this:
Using the ternary operator, you could shorten the expression to:
This example would, of course, return "Old enough."
Multiple Evaluations
You can include multiple evaluations, as well:
Multiple Operations
The ternary operator also allows the inclusion of multiple operations for each expression, separated by a comma:

Ternary Operator Implications
Ternary operators avoid otherwise verbose code , so on the one hand, they appear desirable. On the other hand, they can compromise readability — obviously, "IF ELSE" is more easily understood than a cryptic "?".
When using a ternary operator — or any abbreviation — consider who will be reading your code. If less-experienced developers may need to understand your program logic, perhaps the use of the ternary operator should be avoided. This is especially true if your condition and evaluations are complex enough that you would need to nest or chain your ternary operator. In fact, these kinds of nested operators can impact not only readability but debugging.
As with any programming decision, be sure to consider context and usability before using a ternary operator.
- An Abbreviated JavaScript If Statement
- JavaScript Nested IF/ELSE Statements
- The Dollar Sign ($) and Underscore (_) in JavaScript
- How to Return a Value in JavaScript
- How to Create a Continuous Text Marquee in JavaScript
- How to Create a Continuous Image Marquee With JavaScript
- Moving JavaScript Out of the Web Page
- How to Create and Use External JavaScript Files
- How to Convert Numbers Into Words Using JavaScript
- JavaScript and Emails
- Add the Concentration Memory Game to Your Web Page
- Why JavaScript
- Introduction to JavaScript
- Free JavaScript Download
- Is JavaScript Hard to Learn?
- JavaScript and JScript : What's the Difference?
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Conditional Statements in JavaScript
JavaScript conditional statements allow you to execute specific blocks of code based on conditions. If the condition is met, a particular block of code will run; otherwise, another block of code will execute based on the condition.
There are several methods that can be used to perform Conditional Statements in JavaScript.
Conditional Statement | Description |
---|---|
if statement | Executes a block of code if a specified condition is true. |
else statement | Executes a block of code if the same condition of the preceding if statement is false. |
else if statement | Adds more conditions to the if statement, allowing for multiple alternative conditions to be tested. |
switch statement | Evaluates an expression, then executes the case statement that matches the expression’s value. |
ternary operator | Provides a concise way to write if-else statements in a single line. |
Nested if else statement | Allows for multiple conditions to be checked in a hierarchical manner. |
This table outlines the key characteristics and use cases of each type of conditional statement. Now let’s understand each conditional statement in detail along with the examples.
JavaScript Conditional Statements Examples:
1. using if statement.
The if statement is used to evaluate a particular condition. If the condition holds true, the associated code block is executed.
Example: This JavaScript code determines if the variable `num` is even or odd using the modulo operator `%`. If `num` is divisible by 2 without a remainder, it logs “Given number is even number.” Otherwise, it logs “Given number is odd number.”
2. Using if-else Statement
The if-else statement will perform some action for a specific condition. Here we are using the else statement in which the else statement is written after the if statement and it has no condition in their code block.
Example: This JavaScript code checks if the variable `age` is greater than or equal to 18. If true, it logs “You are eligible for a driving license.” Otherwise, it logs “You are not eligible for a driving license.” This indicates eligibility for driving based on age.
3. else if Statement
The else if statement in JavaScript allows handling multiple possible conditions and outputs, evaluating more than two options based on whether the conditions are true or false.
Example: This JavaScript code determines whether the constant `num` is positive, negative, or zero. If `num` is greater than 0, it logs “Given number is positive.” If `num` is less than 0, it logs “Given number is negative.” If neither condition is met (i.e., `num` is zero), it logs “Given number is zero”.
4. Using Switch Statement (JavaScript Switch Case)
As the number of conditions increases, you can use multiple else-if statements in JavaScript. but when we dealing with many conditions, the switch statement may be a more preferred option.
Example: This JavaScript code assigns a branch of engineering to a student based on their marks. It uses a switch statement with cases for different mark ranges. The student’s branch is determined according to their marks and logged to the console.
5. Using Ternary Operator ( ?: )
The conditional operator, also referred to as the ternary operator (?:), is a shortcut for expressing conditional statements in JavaScript.
Example: This JavaScript code checks if the variable `age` is greater than or equal to 18. If true, it assigns the string “You are eligible to vote.” to the variable `result`. Otherwise, it assigns “You are not eligible to vote.” The value of `result` is then logged to the console.
6. Nested if…else
Nested if…else statements in JavaScript allow us to create complex conditional logic by checking multiple conditions in a hierarchical manner. Each if statement can have an associated else block, and within each if or else block, you can nest another if…else statement. This nesting can continue to multiple levels, but it’s important to maintain readability and avoid excessive complexity.
Example: In this example, the outer if statement checks the weather variable. If it’s “sunny,” it further checks the temperature variable to determine the type of day it is (hot, warm, or cool). Depending on the values of weather and temperature, different messages will be logged to the console.
Similar Reads
- Web Technologies
- javascript-basics
Please Login to comment...
- How to Underline in Discord
- How to Block Someone on Discord
- How to Report Someone on Discord
- How to add Bots to Discord Servers
- GeeksforGeeks Practice - Leading Online Coding Platform
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript if, else, and else if.
Conditional statements are used to perform different actions based on different conditions.
Conditional Statements
Very often when you write code, you want to perform different actions for different decisions.
You can use conditional statements in your code to do this.
In JavaScript we have the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true
- Use else to specify a block of code to be executed, if the same condition is false
- Use else if to specify a new condition to test, if the first condition is false
- Use switch to specify many alternative blocks of code to be executed
The switch statement is described in the next chapter.
The if Statement
Use the if statement to specify a block of JavaScript code to be executed if a condition is true.
Note that if is in lowercase letters. Uppercase letters (If or IF) will generate a JavaScript error.
Make a "Good day" greeting if the hour is less than 18:00:
The result of greeting will be:
Advertisement
The else Statement
Use the else statement to specify a block of code to be executed if the condition is false.
If the hour is less than 18, create a "Good day" greeting, otherwise "Good evening":
The else if Statement
Use the else if statement to specify a new condition if the first condition is false.
If time is less than 10:00, create a "Good morning" greeting, if not, but time is less than 20:00, create a "Good day" greeting, otherwise a "Good evening":
More Examples
Random link This example will write a link to either W3Schools or to the World Wildlife Foundation (WWF). By using a random number, there is a 50% chance for each of the links.
Test Yourself With Exercises
Fix the if statement to alert "Hello World" if x is greater than y .
Start the Exercise
Test Yourself with Exercises!
Exercise 1 » Exercise 2 »

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
javascript: is this a conditional assignment?
From the google analytics tracking code:
how does this work?
Is it a conditional variable value assignment? Is it the same as saying:
- possible duplicate of What does the || operator do? – Felix Kling Commented May 29, 2011 at 13:08
- Short answer: yes (although the second one creates a global _gaq edit: if we assume that _gaq does not exist). – Felix Kling Commented May 29, 2011 at 13:08
- @Felix — unless something else applies a different scope to _gaq – Quentin Commented May 29, 2011 at 13:12
- I would add that given the specifics of this questions, those two statements also differ in that you cannot use the syntax of the former to conditionally define a global variable as it will throw a reference error. – Andrew Hubbs Commented Mar 15, 2012 at 20:26
- Also worth noting is that if you had purposely set _gaq to a falsy value (like 0 ), then it would be reassigned the value of [] . So it doesn't behave exactly like conditional assignment in Ruby. – steve Commented May 10, 2012 at 7:53
4 Answers 4
The or operator ( || ) will return the left hand side if it is a true value, otherwise it will return the right hand side.
It is very similar to your second example, but since it makes use of the var keyword, it also establishes a local scope for the variable.
Yes, it is.
The || operator evaluates to its leftmost "truthy" operand. If _gaq is "falsy" (such as null , undefined , or 0 ), it will evaluate to the right side ( [] ).
- 3 or false or "" (empty string) – Russ Cam Commented May 29, 2011 at 13:15
It's the same as saying:
(This can be done since the var is hoisted above the conditional check, thereby avoiding a 'not defined' error, and it will also cause _gaq to be automatically treated as local in scope.)
Actually it's not the same as saying:
at least not necessarily. Consider this:
When there's a "_gaq" (I hate typing that, by the way) in an outer lexical scope, what you end up with is a new variable in the inner scope. The "if" statement differs in that very important way — there would only be one "_gaq" in that case.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript or ask your own question .
- The Overflow Blog
- Where developers feel AI coding tools are working—and where they’re missing...
- He sold his first company for billions. Now he’s building a better developer...
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Should low-scoring meta questions no longer be hidden on the Meta.SO home...
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- How long have people been predicting the collapse of the family?
- Purpose of sleeve on sledge hammer handle
- What is the simplest formula for calculating the circumference of a circle?
- How can the doctor measure out a dose (dissolved in water) of exactly 10% of a tablet?
- is it okay to mock a database when writing unit test?
- Could you compress chocolate such that it has the same density and shape as a real copper coin?
- God and Law of Identity Paradox
- What should you list as location in job application?
- Can I breed fish in Minecraft?
- Lovecraftian (but not written by Lovecraft himself) horror story featuring a body of water that glowed blue at night due to the creatures in it
- Why is China not mentioned in the Fallout TV series despite its significant role in the games' lore?
- cURL in bash script reads $HOME as /root/
- find command does not work
- Does legislation on transgender healthcare affect medical researchers?
- Double 6x6 Beam
- Does a passenger jet detect excessive weight in the tail after it's just landed?
- Sticky goo making it hard to open and close the main 200amp breaker
- What is the meaning of "I apply my personality in a paste"?
- When can I book German train tickets for January 2025?
- What does mean by "offer accepted" in Mathjobs portal?
- How can moving observer explain non-simultaneity?
- How to Vertically Stack Multiple Plots with Custom Color Functions and Opacity in Mathematica?
- Is there any language which distinguishes between “un” as in “not” and “un” as in “inverse”?
- Let's say Kant was right about Transcendental Idealism, what further conclusions would you come to about reality and our existence?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Remember language
Nullish coalescing assignment (??=)
The nullish coalescing assignment ( ??= ) operator, also known as the logical nullish assignment operator, only evaluates the right operand and assigns to the left if the left operand is nullish ( null or undefined ).
Description
Nullish coalescing assignment short-circuits , meaning that x ??= y is equivalent to x ?? (x = y) , except that the expression x is only evaluated once.
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :
Neither would the following trigger the setter:
In fact, if x is not nullish, y is not evaluated at all.
Using nullish coalescing assignment
You can use the nullish coalescing assignment operator to apply default values to object properties. Compared to using destructuring and default values , ??= also applies the default value if the property has value null .
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Nullish coalescing operator ( ?? )

IMAGES
VIDEO
COMMENTS
There are two methods I know of that you can declare a variable's value by conditions. Method 1: If the condition evaluates to true, the value on the left side of the column would be assigned to the variable. If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into ...
but it contain two or more lines and cannot assign to a variable. Javascript have a solution for this Problem Ternary Operator. Ternary Operator can write in one line and assign to a variable. ... the whole point of the conditional ternary is to shorten conditional assignment values otherwise you should just use an if statement ...
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if...else statement.
Making decisions in your code — conditionals. Overview: JavaScript building blocks. Next. In any programming language, the code needs to make decisions and carry out actions accordingly depending on different inputs. For example, in a game, if the player's number of lives is 0, then it's game over. In a weather app, if it is being looked at ...
In the code above, JavaScript first checks year < 2015. If that is falsy, it goes to the next condition year > 2015. If that is also falsy, it shows the last alert. There can be more else if blocks. The final else is optional. Conditional operator '?' Sometimes, we need to assign a variable depending on a condition. For instance:
In this example, I used the ternary operator to determine whether a user's age is greater than or equal to 18. Firstly, I used the prompt() built-in JavaScript function. This function opens a dialog box with the message What is your age? and the user can enter a value. I store the user's input in the age variable.
Conditional statements control behavior in JavaScript and determine whether or not pieces of code can run. There are multiple different types of conditionals in JavaScript including: "If" statements: where if a condition is true it is used to specify execution for a block of code.
JavaScript if...else Statement. In computer programming, the if...else statement is a conditional statement that executes a block of code only when a specific condition is met. For example, Suppose we need to assign different grades to students based on their scores. If a student scores above 90, assign grade A.
else. statement2. condition. An expression that is considered to be either truthy or falsy. statement1. Statement that is executed if condition is truthy. Can be any statement, including further nested if statements. To execute multiple statements, use a block statement ({ /* ... */ }) to group those statements.
The conditional ternary operator in JavaScript assigns a value to a variable based on some condition and is the only JavaScript operator that takes three operands. The ternary operator is a substitute for an if statement in which both the if and else clauses assign different values to the same field, like so:
JavaScript conditional statements allow you to execute specific blocks of code based on conditions. If the condition is met, a particular block of code will run; otherwise, another block of code will execute based on the condition. ... Destructuring assignment allows us to assign the properties of an array or object to a bunch of variables that ...
JavaScript Operators. Operators are used to assign values, compare values, perform arithmetic operations, and more. There are different types of JavaScript operators: Arithmetic Operators. Assignment Operators. Comparison Operators. Logical Operators. Conditional Operators. Type Operators.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... JavaScript Assignment Operators. Assignment operators assign values to JavaScript variables. Operator Example Same As = x = y: x = y += x += y: x = x + y-= x -= y: x = x - y ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Let's say you have storage object like this. const storage = { a : 10, b : 20, } and you would like to add a prop to this conditionally based on score. const score = 90. You would now like to add prop c:30 to storage if score is greater than 100. If score is less than 100, then you want to add d:40 to storage.
If you just want an inline IF (without the ELSE), you can use the logical AND operator: (a < b) && /*your code*/; If you need an ELSE also, use the ternary operation that the other people suggested. edited Jul 15, 2015 at 20:31. answered Jul 14, 2015 at 3:51. Nahn.
In JavaScript we have the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false.
The logical OR assignment (||=) operator only evaluates the right operand and assigns to the left if the left operand is falsy. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short ...
The assignment (=) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. ... Conditional (ternary) operator; Decrement (--) delete; Destructuring assignment ... Note that the implication of the above is that, contrary to popular misinformation, JavaScript does ...
Short answer: yes (although the second one creates a global _gaq edit: if we assume that _gaq does not exist). - Felix Kling. May 29, 2011 at 13:08. @Felix — unless something else applies a different scope to _gaq. - Quentin. May 29, 2011 at 13:12.
Conditional (ternary) operator; Decrement (--) delete; Destructuring assignment; Division (/) ... This is an idiomatic pattern in JavaScript, but it gets verbose when the chain is long, and it's not safe. ... If you use callbacks or fetch methods from an object with a destructuring assignment, ...
No assignment is performed if the left-hand side is not nullish, due to short-circuiting of the nullish coalescing operator. For example, the following does not throw an error, despite x being const :