TypeError: Assignment to Constant Variable in JavaScript
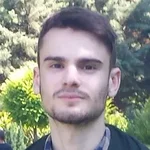
Last updated: Mar 2, 2024 Reading time · 3 min
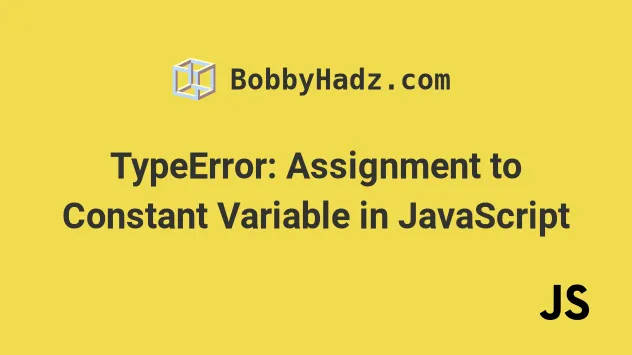

# TypeError: Assignment to Constant Variable in JavaScript
The "Assignment to constant variable" error occurs when trying to reassign or redeclare a variable declared using the const keyword.
When a variable is declared using const , it cannot be reassigned or redeclared.

Here is an example of how the error occurs.
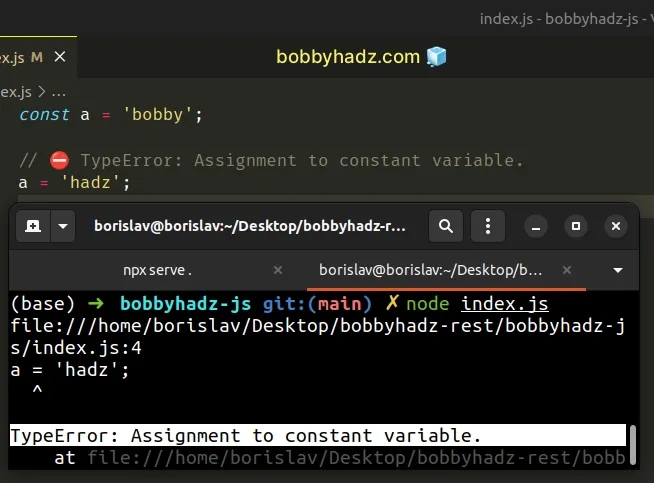
# Declare the variable using let instead of const
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const .
Variables declared using the let keyword can be reassigned.
We used the let keyword to declare the variable in the example.
Variables declared using let can be reassigned, as opposed to variables declared using const .
You can also use the var keyword in a similar way. However, using var in newer projects is discouraged.
# Pick a different name for the variable
Alternatively, you can declare a new variable using the const keyword and use a different name.
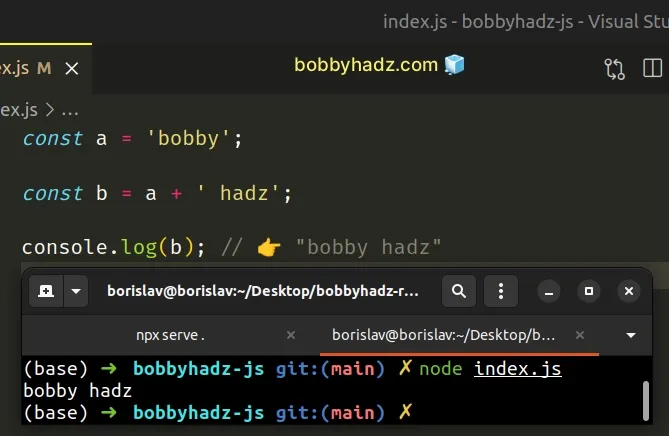
We declared a variable with a different name to resolve the issue.
The two variables no longer clash, so the "assignment to constant" variable error is no longer raised.
# Declaring a const variable with the same name in a different scope
You can also declare a const variable with the same name in a different scope, e.g. in a function or an if block.
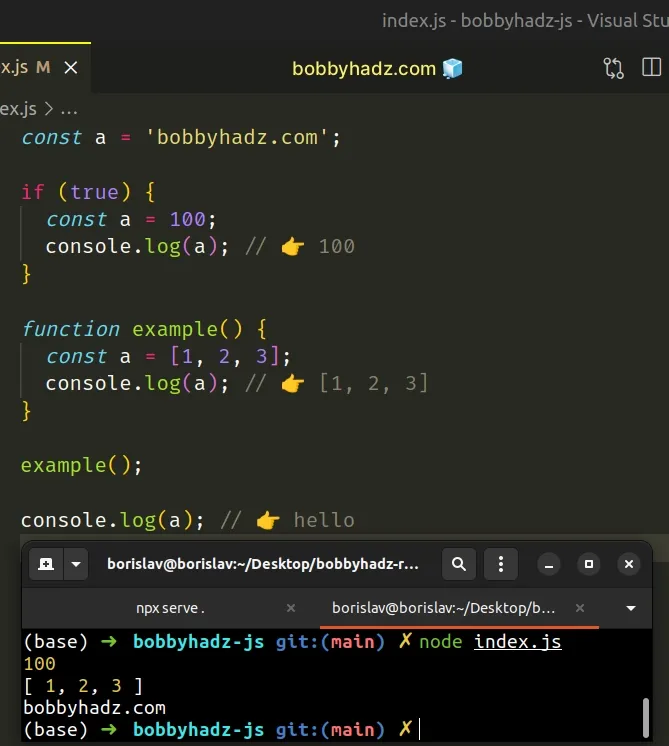
The if statement and the function have different scopes, so we can declare a variable with the same name in all 3 scopes.
However, this prevents us from accessing the variable from the outer scope.
# The const keyword doesn't make objects immutable
Note that the const keyword prevents us from reassigning or redeclaring a variable, but it doesn't make objects or arrays immutable.
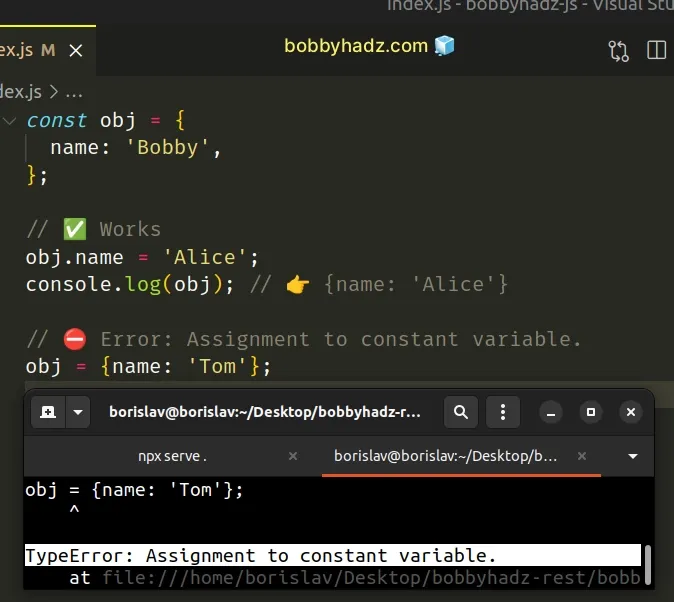
We declared an obj variable using the const keyword. The variable stores an object.
Notice that we are able to directly change the value of the name property even though the variable was declared using const .
The behavior is the same when working with arrays.
Even though we declared the arr variable using the const keyword, we are able to directly change the values of the array elements.
The const keyword prevents us from reassigning the variable, but it doesn't make objects and arrays immutable.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- SyntaxError: Unterminated string constant in JavaScript
- TypeError (intermediate value)(...) is not a function in JS
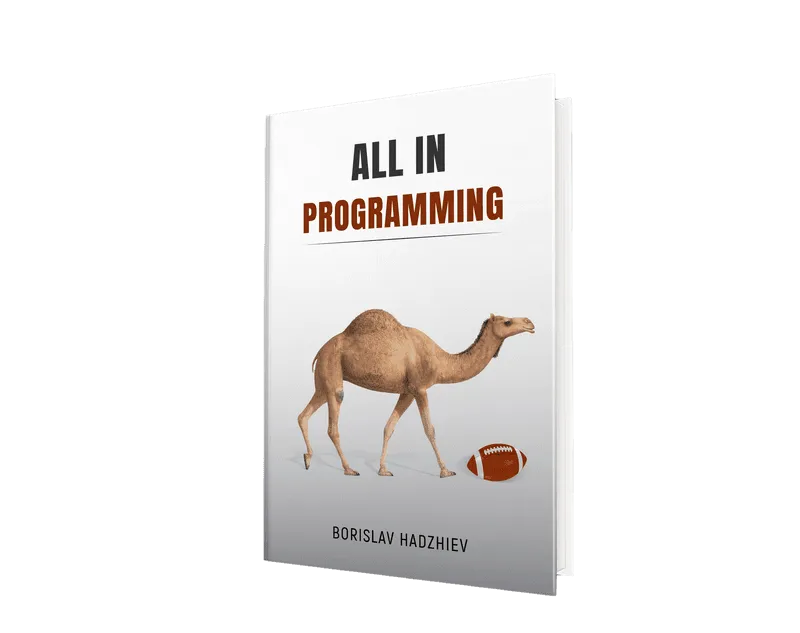
Borislav Hadzhiev
Web Developer
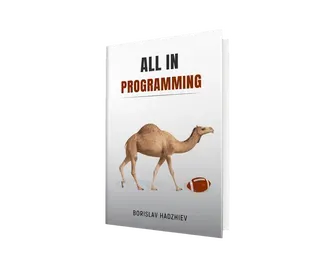
Copyright © 2024 Borislav Hadzhiev
TypeError: Assignment to constant variable when using React useState hook
Abstract: Learn about the common error 'TypeError: Assignment to constant variable' that occurs when using the React useState hook in JavaScript. Understand the cause of the error and how to resolve it effectively.
If you are a React developer, you have probably come across the useState hook, which is a powerful feature that allows you to manage state in functional components. However, there may be times when you encounter a TypeError: Assignment to constant variable error while using the useState hook. In this article, we will explore the possible causes of this error and how to resolve it.
Understanding the Error
The TypeError: Assignment to constant variable error occurs when you attempt to update the value of a constant variable that is declared using the const keyword. In React, when you use the useState hook, it returns an array with two elements: the current state value and a function to update the state value. If you mistakenly try to assign a new value to the state variable directly, you will encounter this error.
Common Causes
There are a few common causes for this error:
- Forgetting to invoke the state update function: When using the useState hook, you need to call the state update function to update the state value. For example, instead of stateVariable = newValue , you should use setStateVariable(newValue) . Forgetting to invoke the function will result in the TypeError: Assignment to constant variable error.
- Using the wrong state update function: If you have multiple state variables in your component, make sure you are using the correct state update function for each variable. Mixing up the state update functions can lead to this error.
- Declaring the state variable inside a loop or conditional statement: If you declare the state variable inside a loop or conditional statement, it will be re-initialized on each iteration or when the condition changes. This can cause the TypeError: Assignment to constant variable error if you try to update the state value.
Resolving the Error
To resolve the TypeError: Assignment to constant variable error, you need to ensure that you are using the state update function correctly and that you are not re-declaring the state variable inside a loop or conditional statement.
If you are forgetting to invoke the state update function, make sure to add parentheses after the function name when updating the state value. For example, change stateVariable = newValue to setStateVariable(newValue) .
If you have multiple state variables, double-check that you are using the correct state update function for each variable. Using the wrong function can result in the error. Make sure to match the state variable name with the corresponding update function.
Lastly, if you have declared the state variable inside a loop or conditional statement, consider moving the declaration outside of the loop or conditional statement. This ensures that the state variable is not re-initialized on each iteration or when the condition changes.
The TypeError: Assignment to constant variable error is a common mistake when using the useState hook in React. By understanding the causes of this error and following the suggested resolutions, you can overcome this issue and effectively manage state in your React applications.
[1] | React Documentation: |
[2] | MDN Web Docs: |
Tags: : javascript reactjs react-state
Latest news
- Fixing Footers with backdrop-filter:blur() and mix-blend-mode:difference in Firefox
- Feign Request Returns 400 Error After Upgrading to Spring Boot 3
- Utilizing Python Script for Large Number Dependencies with AWS Lambda Function
- Python Nanobufferflow: Handling Skipped try-except Blocks with Printed Brackets
- Render Component Instance with h() function in Vuejs
- Understanding Role-Based Access Control with Next-Auth
- Error 401 when Authenticating Python GCP Cloud Functions with Service Account Key
- Automating Data Copypasting between Multiple Worksheets in Excel
- Type Inference Fail in Java: A Look at Foo and Bar Records
- Improving Heatmap Generation with stat_density2d_filled and scale_fill_gradient
- Optimizing a C++ Loop with 12,000 YOLOv8 Detection Results
- Strawberry Perl 5.38.2 on Windows 11: Installing DBD::mysql with MySQL 8.0.37
- Resolving ClassNotFoundException Issue with JInput Library in Java for Game Development
- Resolving Clasp Installation Errors: 'clasp.ps1 not digitally signed' on Windows 10
- Deleting 2 TB MySQL InnoDB Table: Keeping Recent Data
- Batch Converting .mkv to .mp4 with FFmpeg and Subtitle Handling
- XAMPP: Resolving Apache Web Server OpenSSL Certificate Error from Godaddy
- Adding Problem Option Inside Haproxy Backend: A Step-by-Step Guide
- Combining Schema.org Validator Tool and Google: A Guide for Software Developers
- Setting Width for Table Elements: A Two-Dimensional Data Grid Issue with Multiple Lines in Header and Separate Tables
- Using PowerMockito to Test Static Methods: Handling InvocationTargetException
- Implementing Drag-and-Drop Functionality in UITableView: Finder for Folder/Files Hierarchy
- Memory Allocation in C: Changing Pointer Addresses
- Getting Started with Overlaying 1-survcurve Competing Risks Outcome Graphs in R using cmprsk package
- Getting Initial Email Addresses with Non-Standard Formats using JavaScript and MS Graph API
- Maximum Sum Vertices in a Weighted DAG Excluding Directly Connected Vertices: A Vertex Selection Problem
- Dynamically Changing Site Server: Delivering Different Websites Based on Time of Year
- Bash Help: Getting Rid of Leading and Trailing Spaces in Returned String
- Making TypeScript Recognize New Methods Added to Object.prototype
- Parsing Text One Column into Different Columns using T-SQL
- Sequelize: No Rows Returned Given Query
- Formatting Text in Blazor: A Solution to Add Breaks
- Summing Across a Function in R: An Alternative to dplyr's mutate()
- Adding a New Array Entry Inside a Loop Event on Change Input Number in React
- Get MetaData Vector Store Output using Langchain LCELRAGchain: A Step-by-Step Guide
React Tutorial
React hooks, react exercises, react es6 variables.
Before ES6 there was only one way of defining your variables: with the var keyword. If you did not define them, they would be assigned to the global object. Unless you were in strict mode, then you would get an error if your variables were undefined.
Now, with ES6, there are three ways of defining your variables: var , let , and const .
If you use var outside of a function, it belongs to the global scope.
If you use var inside of a function, it belongs to that function.
If you use var inside of a block, i.e. a for loop, the variable is still available outside of that block.
var has a function scope, not a block scope.
let is the block scoped version of var , and is limited to the block (or expression) where it is defined.
If you use let inside of a block, i.e. a for loop, the variable is only available inside of that loop.
let has a block scope.
Get Certified!
const is a variable that once it has been created, its value can never change.
const has a block scope.
The keyword const is a bit misleading.
It does not define a constant value. It defines a constant reference to a value.
Because of this you can NOT:
- Reassign a constant value
- Reassign a constant array
- Reassign a constant object
But you CAN:
- Change the elements of constant array
- Change the properties of constant object

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- React Tutorial
- React Exercise
- React Basic Concepts
- React Components
- React Props
- React Hooks
- React Advanced
- React Examples
- React Interview Questions
- React Projects
- Next.js Tutorial
- React Bootstrap
- React Material UI
- React Ant Design
- React Desktop
- React Rebass
- React Blueprint
- Web Technology
How to declare constant in react class ?
- How to create Class Component in React?
- How to Declare a Constant Variable in JavaScript ?
- How to declare a Constant Variable in PHP?
- How to Define Constants in C++?
- How to create a form in React?
- How to create components in ReactJS ?
- How to create a rating component in ReactJS ?
- How to create a Functional Component in React?
- How to determine the size of a component in ReactJS ?
- How to convert a string to a constant in Ruby?
- How to convert functional component to class component in ReactJS ?
- How to create a Custom Hook in React ?
- How are React Hooks different from class components in ReactJS?
- What are Class Components in React?
- How to implement class constants in TypeScript ?
- How do you initialize state in a class component?
- How to Declare a Variable in JavaScript ?
- How to get single cache data in ReactJS ?
- Different ways to declare variable as constant in C
To declare a constant that can be accessed in a React class component, there are multiple approaches that could be efficiently implemented such that constant is accessible class-wide. Constants can be declared in the following two ways:
- Create a getter method in the class for getting the constant when required.
- Assign the class constant after the declaration of the class.
Create a sample project with the following command:
Now move to the constantDemo folder using the following command:
The Project Structure will look like the following:
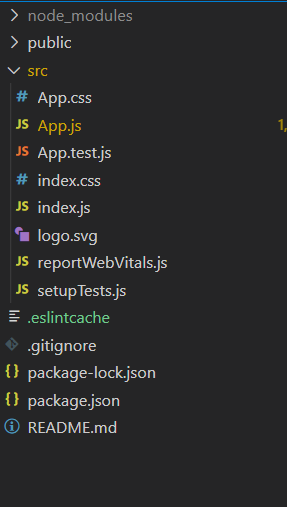
Filename: App.js Now open the App.js file and paste the following code in it:
Now run the project using the following command:
Output :
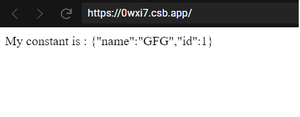
Another way of declaring the constants is shown below. Paste down the following code in the App.js file.
Filename: App.js
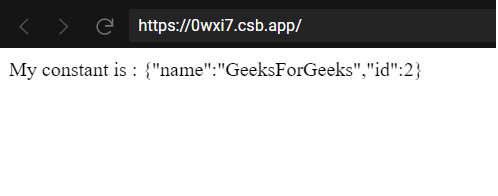
Please Login to comment...
Similar reads.
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How to define constants in React?
In this article, we explain how to define and use constants in React to make your code more maintainable and reusable.
In React, you can define constants using the const keyword. For example:
This creates a constant named MY_CONSTANT with the value "hello". Constants are like variables, except that their value cannot be changed once they are assigned.
You can use constants in React to store values that don't change, such as configuration options or static data that you want to reference in your code.
Here's an example of how you might use a constant in a React component:
In this example, the constant API_ENDPOINT is used to store the URL of an API endpoint. This constant is then used in the fetch call to retrieve data from the API.
It's important to note that constants in React are only available within the scope in which they are defined. In the example above, the MY_CONSTANT constant would only be available within the MyComponent function. If you want to use a constant in multiple components, you would need to define it in a separate file and import it into the components that need it.
Overall, constants can be a useful tool for organizing and managing your code in React. They can help you avoid hardcoding values and make your code more maintainable and reusable.
November 06, 2022
November 03, 2022
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
The const declaration declares block-scoped local variables. The value of a constant can't be changed through reassignment using the assignment operator , but if a constant is an object , its properties can be added, updated, or removed.
The name of the variable to declare. Each must be a legal JavaScript identifier or a destructuring binding pattern .
Initial value of the variable. It can be any legal expression.
Description
The const declaration is very similar to let :
- const declarations are scoped to blocks as well as functions.
- const declarations can only be accessed after the place of declaration is reached (see temporal dead zone ). For this reason, const declarations are commonly regarded as non-hoisted .
- const declarations do not create properties on globalThis when declared at the top level of a script.
- const declarations cannot be redeclared by any other declaration in the same scope.
- const begins declarations , not statements . That means you cannot use a lone const declaration as the body of a block (which makes sense, since there's no way to access the variable). js if ( true ) const a = 1 ; // SyntaxError: Lexical declaration cannot appear in a single-statement context
An initializer for a constant is required. You must specify its value in the same declaration. (This makes sense, given that it can't be changed later.)
The const declaration creates an immutable reference to a value. It does not mean the value it holds is immutable — just that the variable identifier cannot be reassigned. For instance, in the case where the content is an object, this means the object's contents (e.g., its properties) can be altered. You should understand const declarations as "create a variable whose identity remains constant", not "whose value remains constant" — or, "create immutable bindings ", not "immutable values".
Many style guides (including MDN's ) recommend using const over let whenever a variable is not reassigned in its scope. This makes the intent clear that a variable's type (or value, in the case of a primitive) can never change. Others may prefer let for non-primitives that are mutated.
The list that follows the const keyword is called a binding list and is separated by commas, where the commas are not comma operators and the = signs are not assignment operators . Initializers of later variables can refer to earlier variables in the list.
Basic const usage
Constants can be declared with uppercase or lowercase, but a common convention is to use all-uppercase letters, especially for primitives because they are truly immutable.
Block scoping
It's important to note the nature of block scoping.
const in objects and arrays
const also works on objects and arrays. Attempting to overwrite the object throws an error "Assignment to constant variable".
However, object keys are not protected, so the following statement is executed without problem.
You would need to use Object.freeze() to make an object immutable.
The same applies to arrays. Assigning a new array to the variable throws an error "Assignment to constant variable".
Still, it's possible to push items into the array and thus mutate it.
Declaration with destructuring
The left-hand side of each = can also be a binding pattern. This allows creating multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Constants in the JavaScript Guide
Get the Reddit app
A community for discussing anything related to the React UI framework and its ecosystem. Join the Reactiflux Discord (reactiflux.com) for additional React discussion and help.
How come, we can modify the const variable in react but not in vanilla js ?
We use const variables in react and can easily modify the value. While same thing in vanilla js throws error. Why ?
Edit: I'm talking about useState hook. sorry, if am not even asking the right question.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account

error Assignment to constant variable. #2350
rajak9930 commented Feb 19, 2024 • edited Loading
I'm getting a 'resign' error while using 'useRef'. The issue seems to be related to this code: ref={slider => (sliderRef = slider)} import React, { useState, useEffect, useRef } from "react"; |
The text was updated successfully, but these errors were encountered: |
akiran commented Feb 19, 2024 • edited Loading
use instead of for declaring ref |
Sorry, something went wrong.
No branches or pull requests
Typeerror assignment to constant variable
Doesn’t know how to solve the “Typeerror assignment to constant variable” error in Javascript?
Don’t worry because this article will help you to solve that problem
In this article, we will discuss the Typeerror assignment to constant variable , provide the possible causes of this error, and give solutions to resolve the error.
What is Typeerror assignment to constant variable?
“Typeerror assignment to constant variable” is an error message that can occur in JavaScript code.
It means that you have tried to modify the value of a variable that has been declared as a constant.
When we try to reassign greeting to a different value (“Hi”) , we will get the error:
because we are trying to change the value of a constant variable.
How does Typeerror assignment to constant variable occurs ?
In JavaScript, constants are variables whose values cannot be changed once they have been assigned.
Here is an example :
In this example, we declared a constant variable age and assigned it the value 30 .
If you declare an object using the const keyword, you can still modify the properties of the object.
For example:
In this example, we declared a constant object person with two properties ( name and age ).
In this example, we declared a constant variable name and assigned it the value John .
Now let’s fix this error.
Typeerror assignment to constant variable – Solutions
Solution 1: declare the variable using the let or var keyword:.
If you need to modify the value of a variable, you should declare it using the let or var keyword instead of const .
Just like the example below:
Solution 2: Use an object or array instead of a constant variable:
If you need to modify the properties of a variable, you can use an object or array instead of a constant variable.
Solution 3: Declare the variable outside of strict mode:
Solution 4: use the const keyword and use a different name :, solution 5: declare a const variable with the same name in a different scope :.
But with a different value, without modifying the original constant variable.
You can create a new constant variable with the same name, without modifying the original constant variable.
So those are the alternative solutions that you can use to fix the TypeError.
In conclusion, in this article, we discussed “Typeerror assignment to constant variable” , provided its causes and give solutions that resolve the error.
We’re happy to help you.
React - assignment to constant variable using useState()

I am trying to increment slideIndex (which is inside useState() hook) on click event but React throws the following error in the console:
I've tried to change useState() from const to let in my code and it works but I don't think it should be like that:
The problem is that you are trying to increment slideIndex using ++ operator ( ++slideIndex ).
Change ++slideIndex to the slideIndex + 1 and it should work.
Your code should look like this:
Native Advertising
Dirask - we help you to, solve coding problems., ask question..
Go to list of users who liked
More than 3 years have passed since last update.

【React】TypeError: Assignment to constant variableの対処法
下記ソースを実行したときに、TypeError: Assignment to constant variableが発生してしまいました。 翻訳すると、「TypeError: 定数変数への代入」という内容でした。
定数に対して再度値を代入しようとしていたため、起きていたようです。 useEffect内で代入せず、useStateやuseReduverで値を管理するとよさそうです。
Go to list of comments
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
- [email protected]
- 🇮🇳 +91 (630)-411-6234
- Reactjs Development Services
- Flutter App Development Services
- Mobile App Development Services
Web Development
Mobile app development, nodejs typeerror: assignment to constant variable.
Published By: Divya Mahi
Published On: November 17, 2023
Published In: Development
Grasping and Fixing the 'NodeJS TypeError: Assignment to Constant Variable' Issue
Introduction.
Node.js, a powerful platform for building server-side applications, is not immune to errors and exceptions. Among the common issues developers encounter is the “NodeJS TypeError: Assignment to Constant Variable.” This error can be a source of frustration, especially for those new to JavaScript’s nuances in Node.js. In this comprehensive guide, we’ll explore what this error means, its typical causes, and how to effectively resolve it.
Understanding the Error
In Node.js, the “TypeError: Assignment to Constant Variable” occurs when there’s an attempt to reassign a value to a variable declared with the const keyword. In JavaScript, const is used to declare a variable that cannot be reassigned after its initial assignment. This error is a safeguard in the language to ensure the immutability of variables declared as constants.
Diving Deeper
This TypeError is part of JavaScript’s efforts to help developers write more predictable code. Immutable variables can prevent bugs that are hard to trace, as they ensure that once a value is set, it cannot be inadvertently changed. However, it’s important to distinguish between reassigning a variable and modifying an object’s properties. The latter is allowed even with variables declared with const.
Common Scenarios and Fixes
Example 1: reassigning a constant variable.
Javascript:
Fix: Use let if you need to reassign the variable.
Example 2: Modifying an Object's Properties
Fix: Modify the property instead of reassigning the object.
Example 3: Array Reassignment
Fix: Modify the array’s contents without reassigning it.
Example 4: Within a Function Scope
Fix: Declare a new variable or use let if reassignment is needed.
Example 5: In Loops
Fix: Use let for variables that change within loops.
Example 6: Constant Function Parameters
Fix: Avoid reassigning function parameters directly; use another variable.
Example 7: Constants in Conditional Blocks
Fix: Use let if the variable needs to change.
Example 8: Reassigning Properties of a Constant Object
Fix: Modify only the properties of the object.
Strategies to Prevent Errors
Understand const vs let: Familiarize yourself with the differences between const and let. Use const for variables that should not be reassigned and let for those that might change.
Code Reviews: Regular code reviews can catch these issues before they make it into production. Peer reviews encourage adherence to best practices.
Linter Usage: Tools like ESLint can automatically detect attempts to reassign constants. Incorporating a linter into your development process can prevent such errors.
Best Practices
Immutability where Possible: Favor immutability in your code to reduce side effects and bugs. Normally use const to declare variables, and use let only if you need to change their values later .
Descriptive Variable Names: Use clear and descriptive names for your variables. This practice makes it easier to understand when a variable should be immutable.
Keep Functions Pure: Avoid reassigning or modifying function arguments. Keeping functions pure (not causing side effects) leads to more predictable and testable code.
The “NodeJS TypeError: Assignment to Constant Variable” error, while common, is easily avoidable. By understanding JavaScript’s variable declaration nuances and adopting coding practices that embrace immutability, developers can write more robust and maintainable Node.js applications. Remember, consistent coding standards and thorough code reviews are your best defense against common errors like these.
Related Articles
March 13, 2024
Expressjs Error: 405 Method Not Allowed
March 11, 2024
Expressjs Error: 502 Bad Gateway
I’m here to assist you.
Something isn’t Clear? Feel free to contact Us, and we will be more than happy to answer all of your questions.
- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Assignment to constant variable
I try to read the user input and send it as a email. But when I run this code it gives me this error: Assignment to constant variable.

You might want:
I would think the latter option is better, at least stylistically.
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged node.js nodemailer or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- The return of Staging Ground to Stack Overflow
- Policy: Generative AI (e.g., ChatGPT) is banned
- Should we burninate the [lib] tag?
Hot Network Questions
- Is there a tool for generating nanotube from an arbitrary 2D structure?
- New faculty position – expectation to change research direction
- How to make sure to only get full frame lenses for the Canon EF (non-mirrorless) mount?
- Vespertide affairs
- Does "my grades suffered" mean "my grades became worse" or "my grades were bad"?
- Issues with my D&D group
- Is it legal to initialize an array via a functor which takes the array itself as a parameter by reference?
- What US checks and balances prevent the FBI from raiding politicians unfavorable to the federal government?
- Grouping rows by categories avoiding repetition
- Collaborators write their departments for my (undergraduate) affiliation
- Can I replace a GFCI outlet in a bathroom with an outlet having USB ports?
- Do wererats take falling damage?
- How to call up the current value of a pgfkey
- Why does c show up in Schwarzschild's equation for the horizon radius?
- Is FDISK /MBR really undocumented, and why?
- Defining the probability space for rolling a dice infinitely many times
- If a reference is no longer publicly available, should you include the proofs of the results you cite from it?
- Does John 14:13 mean if we literally pray for anything God will act?
- Is "parse out" actually a phrasal verb, and in what context do you use "parse"
- A chess engine in Java: generating white pawn moves
- Short story about soldiers who are fighting against an enemy which turns out to be themselves
- Powering on a laser diode that exceeds PSU max current
- A class for students who want to get better at a subject, aside from their public education
- Proper way to write C code that injects message into /var/log/messages?

IMAGES
VIDEO
COMMENTS
Maybe what you are looking for is Object.assign(resObj, { whatyouwant: value} ). This way you do not reassign resObj reference (which cannot be reassigned since resObj is const), but just change its properties.. Reference at MDN website. Edit: moreover, instead of res.send(respObj) you should write res.send(resObj), it's just a typo
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
To solve the "TypeError: Assignment to constant variable" error, declare the variable using the let keyword instead of using const. Variables declared using the let keyword can be reassigned. The code for this article is available on GitHub. We used the let keyword to declare the variable in the example. Variables declared using let can be ...
For instance, in case the content is an object, this means the object itself can still be altered. This means that you can't mutate the value stored in a variable: js. const obj = { foo: "bar" }; obj = { foo: "baz" }; // TypeError: invalid assignment to const `obj'. But you can mutate the properties in a variable:
In this blog post, we'll explore how to use these new types of variables in your React.js projects. ... // This works fine b = 5; // This also works c = 6; // Error: Assignment to constant variable
TypeError: Assignment to constant variable when using React useState hook If you are a React developer, you have probably come across the useState hook, which is a powerful feature that allows you to manage state in functional components.
It does not define a constant value. It defines a constant reference to a value. Because of this you can NOT: Reassign a constant value; Reassign a constant array; Reassign a constant object; But you CAN: Change the elements of constant array; Change the properties of constant object
In JavaScript, you can declare a constant variable using the const keyword. The const keyword is used to create variables whose values should not be reassigned once they are initially set. Example: Here, pi is a constant variable, and its value is set to 3.14. Once a value is assigned to a constant variable using const, you cannot change that value
In React, you can define constants using the const keyword. For example: const MY_CONSTANT = "hello"; This creates a constant named MY_CONSTANT with the value "hello". Constants are like variables, except that their value cannot be changed once they are assigned. You can use constants in React to store values that don't change, such as ...
TypeError: Assignment to constant variable. System: OSX npm: 6.10.2 node: v10.13. react: 16.8.6. Do you want to request a feature or report a bug? bug What is the current behavior? TypeError: Assignment to constant variable. System: OSX npm: 6.10.2 node: v10.13. react: 16.8.6 ... false in uglify js compress object.
The const declaration creates an immutable reference to a value. It does not mean the value it holds is immutable — just that the variable identifier cannot be reassigned. For instance, in the case where the content is an object, this means the object's contents (e.g., its properties) can be altered. You should understand const declarations as "create a variable whose identity remains ...
React is Javacript, same rules apply. If you attempt. const [val, setVal] = useState(1); val = 3; you get an error, because val is const. - "But I can modify val by calling setVal!" No. By calling setVal you can modify the value stored inside the state (kept inside React, tied to your React component). But you are not changing '' val ' (the ...
import { Link } from "react-router-dom"; import Slider from "react-slick"; const CustomersAry = [ { id: "01", title: ` Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Quis ipsum suspendisse ultrices gravida.
You can create a new constant variable with the same name, without modifying the original constant variable. By declaring a constant variable with the same name in a different scope. This can be useful when you need to use the same variable name in multiple scopes without causing conflicts or errors.
React - assignment to constant variable using useState() 1 answers. 0 points. Asked by: christa ... at onClick (Slider.js:107:1) at HTMLUnknownElement.callCallback (react-dom.development.js:4165:1) at Object.invokeGuardedCallbackDev (react-dom.development.js:4213:1) at invokeGuardedCallback (react-dom.development.js:4278:1) at ...
症状. 下記ソースを実行したときに、TypeError: Assignment to constant variableが発生してしまいました。 翻訳すると、「TypeError: 定数変数への代入」という内容でした。
In Node.js, the "TypeError: Assignment to Constant Variable" occurs when there's an attempt to reassign a value to a variable declared with the const keyword. In JavaScript, const is used to declare a variable that cannot be reassigned after its initial assignment.
What is the best practice in react.js to use a constants value in a component. 0. How to make a variable constant name in React. Hot Network Questions Can a star that initially started with less energy output than the sun (when it was young) evolve to be as luminous as the sun in the same time period
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.