- Standard Template Library
- STL Priority Queue
- STL Interview Questions
- STL Cheatsheet
- C++ Templates
- C++ Functors
- C++ Iterators

std::string::assign() in C++
The member function assign() is used for the assignments, it assigns a new value to the string, replacing its current contents. Syntax 1: Assign the value of string str.
Output:
Syntax 2: Assigns at most str_num characters of str starting with index str_idx. It throws out_of _range if str_idx > str. size().
Syntax 3: Assign the characters of the C-string cstr. It throws length_error if the resulting size exceeds the maximum number of characters.
Syntax 4: Assigns chars_len characters of the character array chars. It throws length_error if the resulting size exceeds the maximum number of characters.
Syntax 5: Assigns num occurrences of character c. It throws length_error if num is equal to string::npos
Syntax 6: Assigns all characters of the range [beg, end). It throws length_error if range outruns the actual content of string.
If you like GeeksforGeeks(We know you do!) and would like to contribute, you can also write an article using write.geeksforgeeks.org or mail your article to [email protected].
Similar Reads
- std::string::append() in C++ The string::append() in C++ is used append the characters or the string at the end of the string. It is the member function of std::string class . The string::append() function can be used to do the following append operations: Table of Content Append a Whole StringAppend a Part of the StringAppend 3 min read
- Setting Bits in C++ Setting a bit in a binary number means changing a specific bit's value to 1. This is a fundamental operation in programming, especially useful in areas like memory management, data processing, and hardware control. In this article, we'll explore how to set a bit at a specific position in a binary nu 4 min read
- Substring in C++ The substring function is used for handling string operations like strcat(), append(), etc. It generates a new string with its value initialized to a copy of a sub-string of this object. In C++, the header file which is required for std::substr(), string functions is <string>. The substring fu 8 min read
- Strings in C++ C++ strings are sequences of characters stored in a char array. Strings are used to store words and text. They are also used to store data, such as numbers and other types of information. Strings in C++ can be defined either using the std::string class or the C-style character arrays. 1. C Style Str 11 min read
- std::stof in C++ Parses string interpreting its content as a floating-point number, which is returned as a value of type float. Syntax : float stof (const string& str, size_t* idx = 0); float stof (const wstring& str, size_t* idx = 0); Parameters : str : String object with the representation of a floating-po 2 min read
- std::string::back() in C++ with Examples This function returns a direct reference to the last character of the string. This shall only be used on non-empty strings. This can be used to access the last character of the string as well as to append a character at the end of the string. Length of the string remains unchanged after appending a 2 min read
- Assignment Operators In C++ In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign 7 min read
- Vector begin() in C++ STL In C++, the vector begin() is a built-in method used to obtain an iterator pointing to the start of the vector. This iterator is used to traverse the vector or perform operations starting from the beginning of the vector. Let’s take a look at an example that illustrates the use of the vector begin() 4 min read
- Understanding static_assert in C++ 11 What is static assertion? Static assertions are a way to check if a condition is true when the code is compiled. If it isn't, the compiler is required to issue an error message and stop the compiling process. The condition that needs to be checked is a constant expression. Performs compile-time asse 6 min read
- getline (string) in C++ The C++ getline() is a standard library function that is used to read a string or a line from an input stream. It is a part of the <string> header. The getline() function extracts characters from the input stream and appends it to the string object until the delimiting character is encountered 5 min read
- Convert String to int in C++ Converting a string to int is one of the most frequently encountered tasks in C++. As both string and int are not in the same object hierarchy, we cannot perform implicit or explicit type casting as we can do in case of double to int or float to int conversion. Conversion is mostly done so that we c 8 min read
- String Functions In C++ A string is referred to as an array of characters. In C++, a stream/sequence of characters is stored in a char array. C++ includes the std::string class that is used to represent strings. It is one of the most fundamental datatypes in C++ and it comes with a huge set of inbuilt functions. In this ar 9 min read
- Move Assignment Operator in C++ 11 In C++ programming, we have a feature called the move assignment operator, which was introduced in C++11. It helps us handle objects more efficiently, especially when it comes to managing resources like memory. In this article, we will discuss move assignment operators, when they are useful and call 5 min read
- STD::array in C++ The array is a collection of homogeneous objects and this array container is defined for constant size arrays or (static size). This container wraps around fixed-size arrays and the information of its size are not lost when declared to a pointer. In order to utilize arrays, we need to include the ar 5 min read
- Stack in C++ STL Stacks are a type of container adaptors with LIFO(Last In First Out) type of working, where a new element is added at one end (top) and an element is removed from that end only. Stack uses an encapsulated object of either vector or deque (by default) or list (sequential container class) as its under 5 min read
- std::any Class in C++ any is one of the newest features of C++17 that provides a type-safe container to store single value of any type. In layman's terms, it is a container which allows one to store any value in it without worrying about the type safety. It acts as an extension to C++ by mimicking behaviour similar to an 6 min read
- Working and Examples of bind () in C++ STL In C++, the std::bind function is a part of the Standard Template Library (STL) and it is used to bind a function or a member function to a specific object or value. It creates a new function object by "binding" a function or member function to a specific object or value, allowing it to be called wi 5 min read
- std::unique in C++ In C++, std::unique is used to remove duplicates of any element present consecutively in a range[first, last). It performs this task for all the sub-groups present in the range having the same element present consecutively. It does not delete all the duplicate elements, but it removes duplicacy by j 7 min read
- std::subtract_with_carry_engine in C++ The std::subtract_with_carry_engine is a random number generator engine provided by the C++ standard library. It is a template class that represents a subtract-with-carry engine which is a type of the random number generator algorithm. It is defined inside <random> header files. Syntaxstd::sub 2 min read
- cpp-strings-library
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Strings in C: How to Declare & Initialize a String Variables in C
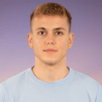
What is String in C?
A String in C is nothing but a collection of characters in a linear sequence. ‘C’ always treats a string a single data even though it contains whitespaces. A single character is defined using single quote representation. A string is represented using double quote marks.
‘C’ provides standard library <string.h> that contains many functions which can be used to perform complicated operations easily on Strings in C.
How to Declare a String in C?
A C String is a simple array with char as a data type. ‘C’ language does not directly support string as a data type. Hence, to display a String in C, you need to make use of a character array.
The general syntax for declaring a variable as a String in C is as follows,
The classic Declaration of strings can be done as follow:
The size of an array must be defined while declaring a C String variable because it is used to calculate how many characters are going to be stored inside the string variable in C. Some valid examples of string declaration are as follows,
The above example represents string variables with an array size of 15. This means that the given C string array is capable of holding 15 characters at most. The indexing of array begins from 0 hence it will store characters from a 0-14 position. The C compiler automatically adds a NULL character ‘\0’ to the character array created.
How to Initialize a String in C?
Let’s study the String initialization in C. Following example demonstrates the initialization of Strings in C,
In string3, the NULL character must be added explicitly, and the characters are enclosed in single quotation marks.
‘C’ also allows us to initialize a string variable without defining the size of the character array. It can be done in the following way,
The name of Strings in C acts as a pointer because it is basically an array.
C String Input: C Program to Read String
When writing interactive programs which ask the user for input, C provides the scanf(), gets(), and fgets() functions to find a line of text entered from the user.
When we use scanf() to read, we use the “%s” format specifier without using the “&” to access the variable address because an array name acts as a pointer. For example:
The problem with the scanf function is that it never reads entire Strings in C. It will halt the reading process as soon as whitespace, form feed, vertical tab, newline or a carriage return occurs. Suppose we give input as “Guru99 Tutorials” then the scanf function will never read an entire string as a whitespace character occurs between the two names. The scanf function will only read Guru99 .
RELATED ARTICLES
- C Tokens, Identifiers, Keywords: What is Tokens & Its Types
- Type Casting in C: Type Conversion, Implicit, Explicit with Example
- calloc() Function in C Library with Program EXAMPLE
- realloc() Function in C Library: How to use? Syntax & Example
In order to read a string contains spaces, we use the gets() function. Gets ignores the whitespaces. It stops
reading when a newline is reached (the Enter key is pressed).
For example:
Another safer alternative to gets() is fgets() function which reads a specified number of characters. For example:
The fgets() arguments are :
- the string name,
- the number of characters to read,
- stdin means to read from the standard input which is the keyboard.
C String Output: C program to Print a String
The standard printf function is used for printing or displaying Strings in C on an output device. The format specifier used is %s
String output is done with the fputs() and printf() functions.
fputs() function
The fputs() needs the name of the string and a pointer to where you want to display the text. We use stdout which refers to the standard output in order to print to the screen. For example:
puts function
The puts function is used to Print string in C on an output device and moving the cursor back to the first position. A puts function can be used in the following way,
The syntax of this function is comparatively simple than other functions.
The string library
The standard ‘C’ library provides various functions to manipulate the strings within a program. These functions are also called as string handlers. All these handlers are present inside <string.h> header file.
Lets consider the program below which demonstrates string library functions:
Other important library functions are:
- strncmp(str1, str2, n) :it returns 0 if the first n characters of str1 is equal to the first n characters of str2, less than 0 if str1 < str2, and greater than 0 if str1 > str2.
- strncpy(str1, str2, n) This function is used to copy a string from another string. Copies the first n characters of str2 to str1
- strchr(str1, c): it returns a pointer to the first occurrence of char c in str1, or NULL if character not found.
- strrchr(str1, c): it searches str1 in reverse and returns a pointer to the position of char c in str1, or NULL if character not found.
- strstr(str1, str2): it returns a pointer to the first occurrence of str2 in str1, or NULL if str2 not found.
- strncat(str1, str2, n) Appends (concatenates) first n characters of str2 to the end of str1 and returns a pointer to str1.
- strlwr() :to convert string to lower case
- strupr() :to convert string to upper case
- strrev() : to reverse string
Converting a String to a Number
In C programming, we can convert a string of numeric characters to a numeric value to prevent a run-time error. The stdio.h library contains the following functions for converting a string to a number:
- int atoi(str) Stands for ASCII to integer; it converts str to the equivalent int value. 0 is returned if the first character is not a number or no numbers are encountered.
- double atof(str) Stands for ASCII to float, it converts str to the equivalent double value. 0.0 is returned if the first character is not a number or no numbers are encountered.
- long int atol(str) Stands for ASCII to long int, Converts str to the equivalent long integer value. 0 is returned if the first character is not a number or no numbers are encountered.
The following program demonstrates atoi() function:
- A string pointer declaration such as char *string = “language” is a constant and cannot be modified.
- A string is a sequence of characters stored in a character array.
- A string is a text enclosed in double quotation marks.
- A character such as ‘d’ is not a string and it is indicated by single quotation marks.
- ‘C’ provides standard library functions to manipulate strings in a program. String manipulators are stored in <string.h> header file.
- A string must be declared or initialized before using into a program.
- There are different input and output string functions, each one among them has its features.
- Don’t forget to include the string library to work with its functions
- We can convert string to number through the atoi(), atof() and atol() which are very useful for coding and decoding processes.
- We can manipulate different strings by defining a array of strings in C.
You Might Like:
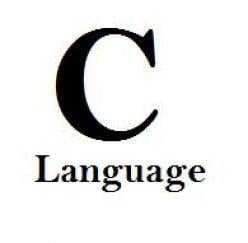
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials.
Created with over a decade of experience.
Certification Courses
Created with over a decade of experience and thousands of feedback.
C Introduction
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
C Programming Strings
String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find the Frequency of Characters in a String
- Sort Elements in Lexicographical Order (Dictionary Order)
- Remove all Characters in a String Except Alphabets
In C programming, a string is a sequence of characters terminated with a null character \0 . For example:
When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default.

- How to declare a string?
Here's how you can declare strings:
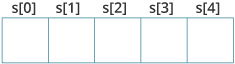
Here, we have declared a string of 5 characters.
- How to initialize strings?
You can initialize strings in a number of ways.
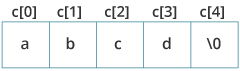
Let's take another example:
Here, we are trying to assign 6 characters (the last character is '\0' ) to a char array having 5 characters. This is bad and you should never do this.
Assigning Values to Strings
Arrays and strings are second-class citizens in C; they do not support the assignment operator once it is declared. For example,
Note: Use the strcpy() function to copy the string instead.
Read String from the user
You can use the scanf() function to read a string.
The scanf() function reads the sequence of characters until it encounters whitespace (space, newline, tab, etc.).
Example 1: scanf() to read a string
Even though Dennis Ritchie was entered in the above program, only "Dennis" was stored in the name string. It's because there was a space after Dennis .
Also notice that we have used the code name instead of &name with scanf() .
This is because name is a char array, and we know that array names decay to pointers in C.
Thus, the name in scanf() already points to the address of the first element in the string, which is why we don't need to use & .
How to read a line of text?
You can use the fgets() function to read a line of string. And, you can use puts() to display the string.

Example 2: fgets() and puts()
Here, we have used fgets() function to read a string from the user.
fgets(name, sizeof(name), stdlin); // read string
The sizeof(name) results to 30. Hence, we can take a maximum of 30 characters as input which is the size of the name string.
To print the string, we have used puts(name); .
Note: The gets() function can also be to take input from the user. However, it is removed from the C standard. It's because gets() allows you to input any length of characters. Hence, there might be a buffer overflow.
Passing Strings to Functions
Strings can be passed to a function in a similar way as arrays. Learn more about passing arrays to a function .
Example 3: Passing string to a Function
Strings and pointers.
Similar like arrays, string names are "decayed" to pointers. Hence, you can use pointers to manipulate elements of the string. We recommended you to check C Arrays and Pointers before you check this example.
Example 4: Strings and Pointers
Commonly used string functions.
- strlen() - calculates the length of a string
- strcpy() - copies a string to another
- strcmp() - compares two strings
- strcat() - concatenates two strings
Table of Contents
- Read and Write String: gets() and puts()
- Passing strings to a function
- Strings and pointers
- Commonly used string functions
Before we wrap up, let’s put your knowledge of C Programming Strings to the test! Can you solve the following challenge?
Write a function to check if a given string is empty or not.
- Return 1 if the string is empty, otherwise, return 0 .
- For example, if str = "Hello" , the expected output is 0
Video: C Strings
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials

22.5 — std::string assignment and swapping
String assignment
The easiest way to assign a value to a string is to use the overloaded operator= function. There is also an assign() member function that duplicates some of this functionality.
The assign() member function also comes in a few other flavors:
If you have two strings and want to swap their values, there are two functions both named swap() that you can use.
C Functions
C structures, c reference.
Strings are used for storing text/characters.
For example, "Hello World" is a string of characters.
Unlike many other programming languages, C does not have a String type to easily create string variables. Instead, you must use the char type and create an array of characters to make a string in C:
Note that you have to use double quotes ( "" ).
To output the string, you can use the printf() function together with the format specifier %s to tell C that we are now working with strings:
Access Strings
Since strings are actually arrays in C, you can access a string by referring to its index number inside square brackets [] .
This example prints the first character (0) in greetings :
Note that we have to use the %c format specifier to print a single character .
Modify Strings
To change the value of a specific character in a string, refer to the index number, and use single quotes :
Advertisement
Loop Through a String
You can also loop through the characters of a string, using a for loop:
And like we specified in the arrays chapter, you can also use the sizeof formula (instead of manually write the size of the array in the loop condition (i < 5) ) to make the loop more sustainable:
Another Way Of Creating Strings
In the examples above, we used a "string literal" to create a string variable. This is the easiest way to create a string in C.
You should also note that you can create a string with a set of characters. This example will produce the same result as the example in the beginning of this page:
Why do we include the \0 character at the end? This is known as the "null terminating character", and must be included when creating strings using this method. It tells C that this is the end of the string.
Differences
The difference between the two ways of creating strings, is that the first method is easier to write, and you do not have to include the \0 character, as C will do it for you.
You should note that the size of both arrays is the same: They both have 13 characters (space also counts as a character by the way), including the \0 character:
Real-Life Example
Use strings to create a simple welcome message:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
cppreference.com
Std::basic_string<chart,traits,allocator>:: assign.
Replaces the contents of the string.
- If only t is provided, replaces the contents with all characters in sv .
- If count is npos , replaces the contents with all characters in sv starting from pos .
- Otherwise, replaces the contents with the std:: min ( count, sv. size ( ) - pos ) characters in sv starting from pos .
- std:: is_convertible_v < const SV & , std:: basic_string_view < CharT, Traits >> is true .
- std:: is_convertible_v < const SV & , const CharT * > is false .
- If count is npos , replaces the contents with all characters in str starting from pos .
- Otherwise, replaces the contents with the std:: min ( count, str. size ( ) - pos ) characters in str starting from pos .
[ edit ] Parameters
[ edit ] return value, [ edit ] exceptions.
propagate_on_container_move_assignment :: value ||
If the operation would cause size() to exceed max_size() , throws std::length_error .
If an exception is thrown for any reason, this function has no effect ( strong exception safety guarantee ).
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- conditionally noexcept
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 17 December 2024, at 23:14.
- Privacy policy
- About cppreference.com
- Disclaimers

- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
class templates
- basic_string
- char_traits
- C++11 u16string
- C++11 u32string
- C++11 stold
- C++11 stoll
- C++11 stoul
- C++11 stoull
- C++11 to_string
- C++11 to_wstring
- string::~string
- string::string
member functions
- string::append
- string::assign
- C++11 string::back
- string::begin
- string::c_str
- string::capacity
- C++11 string::cbegin
- C++11 string::cend
- string::clear
- string::compare
- string::copy
- C++11 string::crbegin
- C++11 string::crend
- string::data
- string::empty
- string::end
- string::erase
- string::find
- string::find_first_not_of
- string::find_first_of
- string::find_last_not_of
- string::find_last_of
- C++11 string::front
- string::get_allocator
- string::insert
- string::length
- string::max_size
- string::operator[]
- string::operator+=
- string::operator=
- C++11 string::pop_back
- string::push_back
- string::rbegin
- string::rend
- string::replace
- string::reserve
- string::resize
- string::rfind
- C++11 string::shrink_to_fit
- string::size
- string::substr
- string::swap
member constants
- string::npos
non-member overloads
- getline (string)
- operator+ (string)
- operator<< (string)
- >/" title="operator>> (string)"> operator>> (string)
- relational operators (string)
- swap (string)
std:: string ::assign
Return value, iterator validity, exception safety.

IMAGES
COMMENTS
The first struct is a character array [] and the second struct is a pointer * to the character string (size 8 bytes for a 64-bit machine). According to Stephen Kochan's book "Programming in C", the only time that C lets you assign a constant string is when defining and initializing a char array as in. char name[20] = { "John Doe" }; not even with
Oct 11, 2024 · The C String is stored as an array of characters. The difference between a character array and a C string is that the string in C is terminated with a unique character ‘\0’. C String Declaration Syntax. Declaring a string in C is as simple as declaring a one-dimensional array. Below is the basic syntax for declaring a string.
Oct 28, 2020 · The member function assign() is used for the assignments, it assigns a new value to the string, replacing its current contents. Syntax 1: Assign the value of string str. string& string::assign (const string& str) str : is the string to be assigned. Returns : *this C/C++ Code // CPP code for
Aug 8, 2024 · The name of Strings in C acts as a pointer because it is basically an array. C String Input: C Program to Read String. When writing interactive programs which ask the user for input, C provides the scanf(), gets(), and fgets() functions to find a line of text entered from the user.
In C programming, a string is a sequence of characters terminated with a null character \0. For example: char c[] = "c string"; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default. Memory Diagram
Sep 16, 2022 · string& string::assign (const string& str, size_type index, size_type len) Assigns a substring of str, starting from index, and of length len Throws an out_of_range exception if the index is out of bounds Returns *this so it can be “chained”. Sample code:
Strings. Strings are used for storing text/characters. For example, "Hello World" is a string of characters. Unlike many other programming languages, C does not have a String type to easily create string variables. Instead, you must use the char type and create an array of characters to make a string in C:
Dec 17, 2024 · pointer to a character string to use as source to initialize the string with t object (convertible to std::basic_string_view ) to initialize the characters of the string with
Assigns a new value to the string, replacing its current contents. (1) string Copies str. (2) substring Copies the portion of str that begins at the character position subpos and spans sublen characters (or until the end of str, if either str is too short or if sublen is string::npos).
Aug 6, 2024 · Creating a String. In C, strings are simply arrays of characters. Here's how you can create a string: char greet [] = "Hello"; In this example, greet is a string variable that contains the text "Hello". Coding Task. Create a string named fruit and assign the value "Apple" to it. Try it on CodeChef. Printing Strings. To print a string in C, we ...